Kotlin Chat Messaging Tutorial
How to Build In-App Messaging with Kotlin or Java.
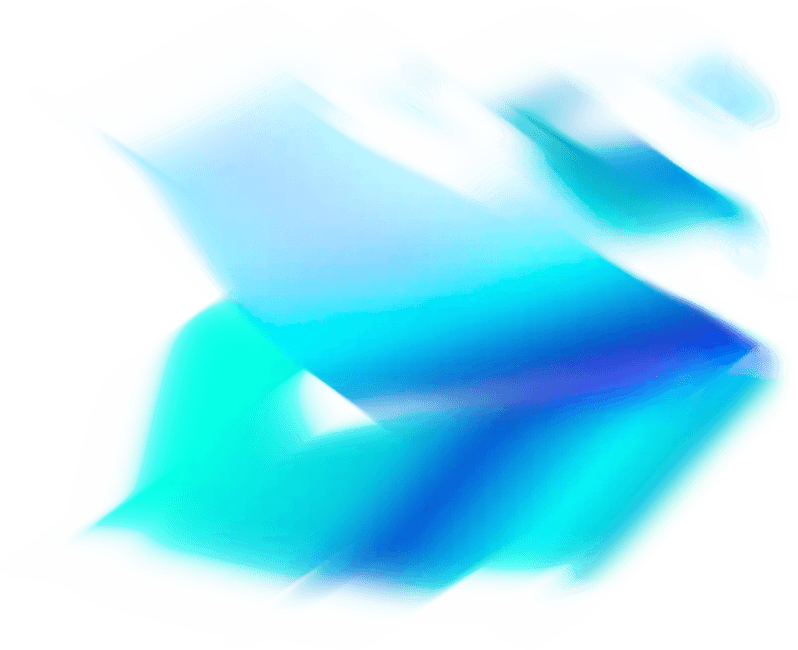
Creating a Project
Confused about "Creating a Project"?
Let us know how we can improve our documentation:
Already all-in on Jetpack Compose? Check out our Compose tutorial instead of this one!
The completed app for each step of the tutorial is available on GitHub.
To get started with the Android Chat SDK, open Android Studio and create a new project.
- Select the
Empty Views Activity
template - Name the project
ChatTutorial
- Set the package name to
com.example.chattutorial
- Select your language - Kotlin (recommended) or Java
- Set the Minimum SDK to 21 (or higher)
- JVM targets 11 (or higher)
Make sure you're using a Material theme in your app
If you're using an up-to-date version of Android Studio, your newly created project should already be using a Theme.MaterialComponents
theme as the parent to its app theme (you can check this in styles.xml
or themes.xml
). If you're running an older version, change the parent theme to be a Material theme instead of Theme.AppCompat
.
If you want to keep using AppCompat theming, you can use a Bridge Theme instead.
Our SDKs are available from MavenCentral, with some of our dependencies being hosted on Jitpack. Update your repositories in the settings.gradle
file like so:
First, we'll enable View Binding. Next, we're going to add the Stream Chat SDK and Coil to our project dependencies. Open up the app module's build.gradle
script and make the following changes:
After you edit your Gradle files, make sure to sync the project (Android Studio will prompt you for this) with the new changes.
Displaying a List of Channels
Confused about "Displaying a List of Channels"?
Let us know how we can improve our documentation:
Stream provides a low-level client, an offline support library, and convenient UI components to help you quickly build your messaging interface. In this section, we'll be using the UI components to quickly display a channel list.
First, open up activity_main.xml
, and change the contents of the file to the following to display a full screen ChannelListView
:
Next, open up MainActivity
and replace the file's contents with the following code:
Let's have a quick look at the source code shown above:
- Step 1: We create a
StreamOfflinePluginFactory
to provide offline support. TheOfflinePlugin
class employs a new caching mechanism powered by side-effects we applied toChatClient
functions. - Step 2: We create a connection to Stream by initializing the
ChatClient
using an API key. This key points to a tutorial environment, but you can sign up for a free Chat trial to get your own later. Next, we add theofflinePluginFactory
to theChatClient
withwithPlugin
method for providing offline storage capabilities. For a production app, we recommend initializing thisChatClient
in your Application class. - Step 3: We create a
User
instance and pass it to theChatClient
'sconnectUser
method, along with a pre-generated user token, in order to authenticate the user. In a real-world application, your authentication backend would generate such a token at login / signup and hand it over to the mobile app. For more information, see the Tokens & Authentication page. - Step 4: We configure the
ChannelListViewModelFactory
with a filter and a sort option. We’re using the default sort option which orders the channels bylast_updated_at
time, putting the most recently used channels on the top. For the filter, we’re specifying all channels of typemessaging
where the current user is a member. The documentation about Querying Channels covers this in more detail. - Step 5: We bind our ChannelListView to the ChannelListViewModel by calling the
bindView
function.
Build and run your application - you should see the channel list interface shown on the right.
Creating a Chat Experience
Confused about "Creating a Chat Experience"?
Let us know how we can improve our documentation:
Next, let's open up one of these channels and start chatting. To do this, we'll leverage the MessageListHeaderView, MessageListView, and MessageComposerView components.
Although our default components provide a robust experience, it's possible to configure and customize them, or even use your own custom views.
Create a new Empty Views Activity (New -> Activity -> Empty Views Activity) and name it ChannelActivity
.
Make sure that ChannelActivity
is added to your manifest. Android Studio does this automatically if you use the wizard to create the Activity, but you'll need to add it yourself if you manually created the Activity class.
Open up activity_channel.xml
and change the layout to the following:
Next, replace the code in ChannelActivity
with this code:
Configuring ChannelActivity
involves a few steps, so let's review what's going on.
- Step 1: We set up three ViewModels:
- MessageListHeaderViewModel - Provides useful information about the channel.
- MessageListViewModel - Loads a channel's messages, while also providing useful information about the current state of the channel.
- MessageComposerViewModel - Responsible for composing and sending new messages.
- Step 2: We bind these
ViewModels
to their respective Views. This loose coupling between components makes it easy to customize things, or only use the components you find necessary. - Steps 3 and 4: We coordinate the
MessageListView
with bothMessageListHeaderView
andMessageComposerView
. TheMessageComposerView
needs to know when you’re editing a message or when you enter a message thread, which is also a piece of useful information forMessageListHeaderView
. - Steps 5 and 6: We create a back button handler, and set the same behavior for the
MessageListHeaderView
and the Activity'sOnBackPressedDispatcher
. The handler sends aBackButtonPressed
event to theMessageListViewModel
, which will decide how to handle this event. If we're in a message thread, it'll navigate back to the channel. If we're already in the channel, it will navigate to the channel list by emitting aNavigateUp
state that we handle by finishingChannelActivity
.
Lastly, we want to launch ChannelActivity
when you tap a channel in the channel list. Open MainActivity
and replace the TODO at the end of the onCreate
method:
If you run the application and tap on a channel, you'll now see the chat interface shown on the right.
Chat Features
Confused about "Chat Features"?
Let us know how we can improve our documentation:
Congrats on getting your chat experience up and running! Stream Chat provides you with all the features you need to build an engaging messaging experience:
- Offline support: send messages, edit messages and send reactions while offline
- Link previews: generated automatically when you send a link
- Commands: type
/
to use commands like/giphy
- Reactions: long-press on a messages to add a reaction
- Attachments: use the paperclip button in
MessageComposerView
to attach images and files - Edit message: long-press on your message for message options, including editing
- Threads: start message threads to reply to any message
You should also notice that, regardless of whether you chose to develop your app in Kotlin or Java, the chat loads very quickly. Stream’s API is powered by Go, RocksDB and Raft. The API tends to respond in less than 10ms and powers activity feeds and chat for over a billion end users.
Some of the features are hard to see in action with just one user online. You can open the same channel on the web and try user-to-user interactions like typing events, reactions, and threads.
Chat Message Customization
Confused about "Chat Message Customization"?
Let us know how we can improve our documentation:
You now have a fully functional mobile chat interface. Not bad for a couple minutes of work! Maybe you'd like to change things up a bit though? No problem! Here are four ways to customize your chat experience:
- Style the
MessageListView
using attributes (easy) - Create a custom attachment view (easy)
- Build your own views on top of the LiveData objects provided by the offline support library (advanced)
- Use the low level client to directly interact with the API
In the next sections, we'll show an example for each type of customization. We'll start by changing the colors of the chat messages to match your theme.
Open activity_channel.xml
and customize the MessageListView
with the following attributes for a green message style:
If you run the app and write a message, you'll notice that messages written by you are now green. The documentation for MessageListView details all the available customization options.
Creating Custom Attachment
Confused about "Creating Custom Attachment"?
Let us know how we can improve our documentation:
There may come a time when you have requirements to include things in your chat experience that we don't provide out-of-the-box. For this purpose, we provide two main customization paths: you can either reimplement the entire ViewHolder
and display a message how you like, or you can use custom attachment views, which is a lot less work. We'll look at this latter approach now.
You could use this to embed a shopping cart in your chat, share a location, or perhaps implement a poll. For this example, we'll keep it simple and customize the preview for images shared from Imgur. We're going to render the Imgur logo over images from the imgur.com domain.
As a first step, download the Imgur logo and add it to your drawable
folder.
Next, create a new layout file called attachment_imgur.xml
:
Now we need to create a custom implementation of AttachmentFactory
. Create a new file called ImgurAttachmentFactory
and add this code:
Let's break down what we're doing above:
- In
canHandle
, we check whether there's an Imgur attachment in the current message. Link previews in the Chat SDK are added to the message as attachments. - If there is an Imgur attachment in the current message,
createViewHolder
will create theImgurAttachmentViewHolder
that inflates the custom Imgur layout, adds some styling (rounded corners), and then loads the Imgur image from the attachment's URL into the containedImageView
. We return this newly created View from the factory, and it'll be added to the message's UI.
Finally, we'll provide an instance of this AttachmentFactoryManager
, which includes the ImgurAttachmentFactory
to the MessageListView
component. Open ChannelActivity
and replace the TODO comment with the following:
Your Custom Attachment View
Confused about "Your Custom Attachment View"?
Let us know how we can improve our documentation:
When you run your app, you should now see the Imgur logo displayed over images from Imgur. You can test this by posting an Imgur link like this one: https://imgur.com/gallery/ro2nIC6
This was, of course, a very simple change, but you could use the same approach to implement a product preview, shopping cart, location sharing, polls, and more. You can achieve lots of your message customization goals by implementing a custom attachment View.
If you need even more customization, you can also implement custom ViewHolders for the entire message object.
Creating a Typing Status Component
Confused about "Creating a Typing Status Component"?
Let us know how we can improve our documentation:
If you want to build a custom UI, you can do that using the StateFlow
objects provided by our offline support library, or the events provided by our low level client. The example below will show you how to build a custom typing status component using both approaches.
First, open activity_channel.xml
and add the following TextView
above the MessageListView
. You'll also want to update the constraints for the MessageListView
.
Option 1 - Typing Status Using the Offline Library
Confused about "Option 1 - Typing Status Using the Offline Library"?
Let us know how we can improve our documentation:
The offline support library enables you to watch a channel and fetch an instance of ChannelState
, which provides observable StateFlow
objects for a channel such as the current user, typing state, reads statuses, etc. The full list of StateFlow
objects provided is detailed in the documentation. These make it easy to obtain data for use in your own custom UI.
Open ChannelActivity
and add the following code below Step 6, still within the onCreate
method (and an additional helper method for Java users):
Remember to update your imports before running the app. You should now see a small typing indicator bar just below the channel header. Note that the current user is excluded from the list of typing users.
The code is quite simple - We watch the channel in order to get ChannelState
, which contains an observable StateFlow
called typing
that provides us typing events.
If you're using Java, you'll notice an additional step that requires transforming StateFlow
s into LiveData
. Coroutines are not commonly used in Java code so here we transform them into LiveData
early to keep the codebase more familiar.
To test the behaviour, you can open a client on the web, enter the same channel, and then type away!
Option 2 - Typing Status Using the Low-Level Client
Confused about "Option 2 - Typing Status Using the Low-Level Client"?
Let us know how we can improve our documentation:
The low-level client enables you to talk directly to Stream's API. This gives you the flexibility to implement any messaging UI that you want. In this case, we want to show who is typing, current user included.
The entry point for the low-level client's APIs is the ChatClient
class. In the code below, we get the ChatClient
instance, and fetch a ChannelClient
using the channel(cid)
call. This provides access to all operations on the given channel.
Then, we use subscribeFor
to listen to all TypingStart
and TypingStop
events in the current channel, and update the contents of the TextView
with the list of typing users. Note that we specify the current Activity
as the lifecycle owner to ensure that the event callbacks are removed when the Activity
is no longer active.
Run your app and start typing in the MessageComposerView
: you'll notice that the typing header on top updates to show that the current user is typing.
You can also use a web client to enter the same channel and generate typing events.
Congratulations!
Confused about "Congratulations!"?
Let us know how we can improve our documentation:
In this Android in-app messaging tutorial, you learned how to build a fully functional chat app using Java or Kotlin. You also learned how easy it is to customize the behavior and build any type of chat or messaging experience.
Remember, you can also check out the completed app for the tutorial on GitHub.
If you want to get started on integrating chat into your own app, sign up for a free Chat trial, and get your own API key to build with!
To recap, our Android Chat SDK consists of three libraries which give you an opportunity to interact with Stream Chat APIs on a different level:
- stream-chat-android-client - The official low-level Android SDK for Stream Chat. It allows you to make API calls and receive events whenever something changes on a user or channel that you’re watching.
- stream-chat-android-offline - Builds on top of the low-level client, adds seamless offline support, optimistic UI updates (great for performance) and exposes StateFlow objects. If you want to build a fully custom UI, this library is your best starting point.
- stream-chat-android-ui-components - Provides ready-to-use UI components while also taking advantage of the offline library and low level SDK. This allows you to ship chat in your application in a matter of days.
The underlying chat API is based on Go, RocksDB, and Raft. This makes the chat experience extremely fast with response times that are often below 10ms.
Final Thoughts
In this chat app tutorial we built a fully functioning Android messaging app with our Android SDK component library. We also showed how easy it is to customize the behavior and the style of the Android chat app components with minimal code changes.
Both the chat SDK for Android and the API have plenty more features available to support more advanced use-cases such as push notifications, content moderation, rich messages and more. Please check out our Flutter tutorial too. If you want some inspiration for your app, download our free chat interface UI kit.
Give us Feedback!
Did you find this tutorial helpful in getting you up and running with Android for adding chat to your project? Either good or bad, we’re looking for your honest feedback so we can improve.
We’ve been going at lightspeed to build more communication functionality into Kiddom. The only way to achieve this in four months was to do a chat integration with Stream because we needed to do it reliably and at scale.
Nick Chen
Head of Product, Kiddom