iOS Activity Feed Components
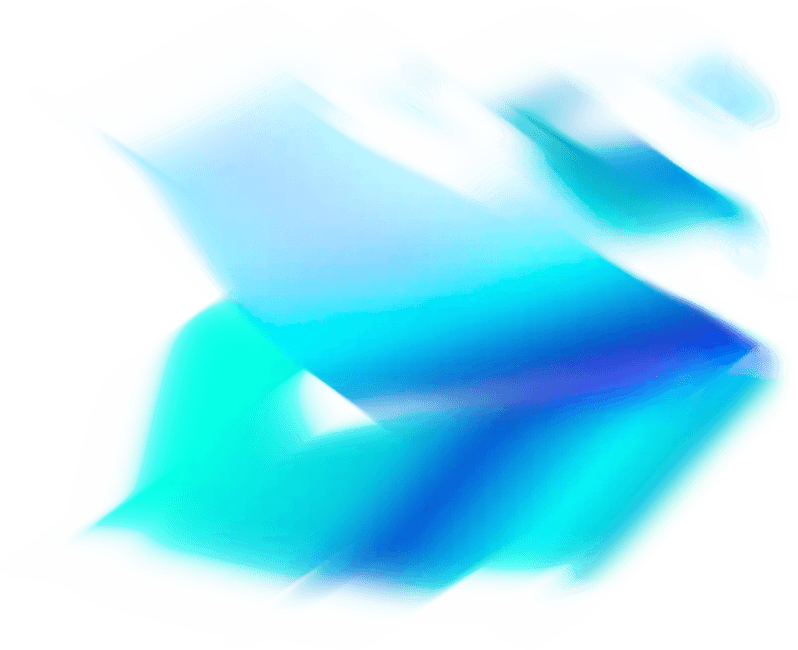
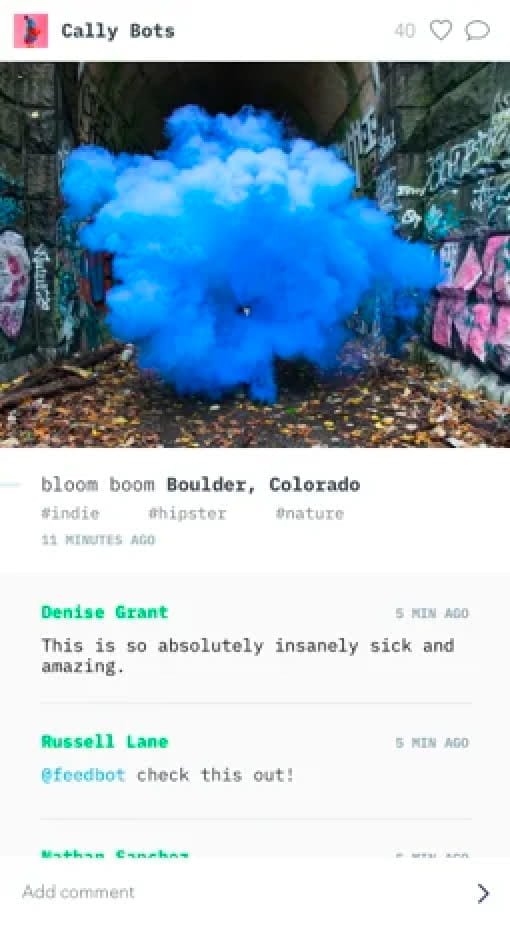
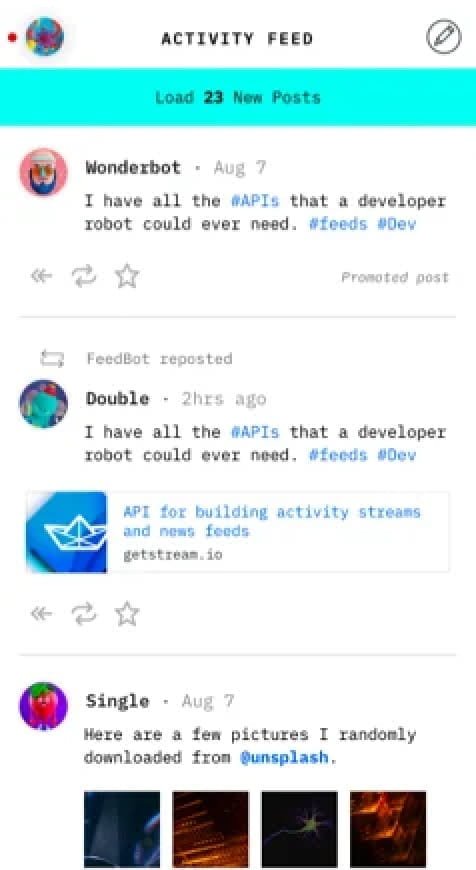
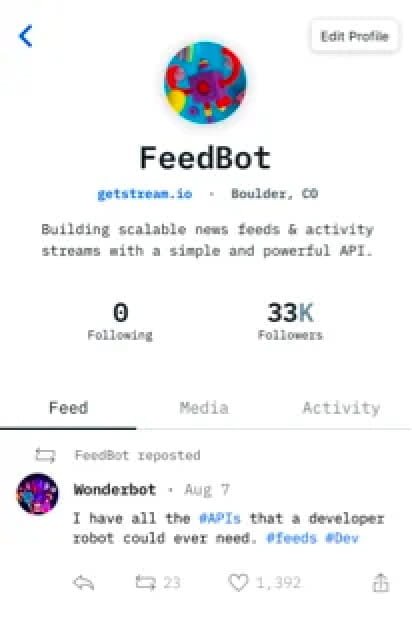
Setup
Confused about "Setup"?
Let us know how we can improve our documentation:
To get started, create a new Swift project in Xcode with a “Single View App” using the project name GetStreamActivityFeedDemo. Stream’s iOS Activity Feed Components are published on all major package repositories; so we’ll use CocoaPods as our dependency manager for this tutorial.
Ensure CocoaPods is installed
Note: If you do not currently have CocoaPods installed, you can do so by running the following command:
Moving On
Now that you are in your project directory and have ensured that CocoaPods is installed, let’s go ahead and initialize CocoaPods and install the Stream Activity Feed Components within our project.
Initialize CocoaPods and create a Podfile:
Open the generated Podfile and modify the contents of the file with the following:
Now that we’ve modified our Podfile, let’s go ahead and install the project dependencies via the terminal with one simple command:
The above command will generate the GetStreamActivityFeedDemo.xcworkspace file automatically. With our workspace now containing our Pods project with dependencies, as well as our original project, let’s go ahead and move over to Xcode to complete the process.
Debugging
If you encounter any issues while installing the package, please make sure that you are using the latest version of CocoaPods and that your repository is up to date. You can use the following command to update:
Note: Occasionally, it helps to clean the CocoaPods cache using the following command:
Once you’ve cleared the CocoaPods cache, it’s important to run the command pod install from within your directory to regenerate the cache.
Add Stream to your application
Confused about "Add Stream to your application"?
Let us know how we can improve our documentation:
To setup Stream we need to add a couple things in your AppDelegate.swift. Here is what your AppDelegate should look like:
Note: You will get a compiler error No such module 'GetStream', it’s expected before building. Build the project using Command+B or ignore the error for now, we’ll build the project before running anyway.
Your application is now configured with the API credentials for the demo application.
Client.shared is a singleton we define in app delegate and re-use throughout the entire application. The shared client manages current user's session (token) and your Stream application API credentials (apiKey and appId).
Note for the impatient: we still need to add a tiny bit of code before we can launch our application. Bear with us for a minute :-)
Because this is a tutorial, we do not have a real signup flow and we will use a pre-generated user session token, on a real-world application, your authentication backend would generate such token at log-in / signup and hand it over to the mobile app.
This is how you would generate the token server side (no need to do this for this tutorial):
Timeline Feed
Confused about "Timeline Feed"?
Let us know how we can improve our documentation:
Adding a timeline feed is very simple, the library comes with a built-in FlatFeedViewController class which loads the current user's timeline using the APIs and renders its content.
Because we started this project as a “Single View App” we already have a ViewController in our project.
The example newsfeed is loaded with some demo data, this way you can see how different kind of activities are rendered out of the box.
Update ViewController
First, we need to update our ViewController
- Open ViewController.swift and import GetStreamActivityFeed and GetStream
- Change ViewController's parent class from the UIViewController to FlatFeedViewController<Activity>
Our application is now showing the content of our user's timeline. To make this more interesting we have added some random example data.
The timeline feed supports out of the box:
- Custom Activity type
- Attachments with images and Open Graph data
- Open web links
- Open an image gallery
- Emojis 🙌
- Pagination
- Refresh by pull from the top
Note: real applications have more than just one view controller and often need more complex customizations. Under the hood the activity feed library allows you fine tune how feeds are used in your application. At the end of the tutorial you can find the link to our example application which shows how to use this library in a real world application.
Likes
Confused about "Likes"?
Let us know how we can improve our documentation:
The Activity Feed Components provide functionality to add/remove reactions to the activities such as likes and comments.
Adding likes to activities is very simple and supported out-of-the-box by the library. The only thing we need to do is change how we initialize the feed presenter class to show likes.
Behind the scenes this adds an additional table view cell o display the Like button and the likes count.
Additional setup for like buttons possible with overriding the next method:
Comments
Confused about "Comments"?
Let us know how we can improve our documentation:
Comments are a very common functionality used on newsfeeds. The library comes with built-in support for comments, let’s see how we can add comments along with each activity.
For this we are going to add a detail screen for each activity. The detail screen will show the full activity information as well as the list of comments and a comment box to post new ones.
First we are going to add this method to our View Controller class:
The code that we just added does two things:
- It handles the tap on activities by overriding tableView’s didSelectRowAt method
- Initializes and present DetailViewController with current activity’s data
As a final touch, we are going to add the comment count next to the like button. For this we only need to add .comments to the list of reactionTypes
This is how the ViewController should look after these two changes:
Bonus points: our library also supports nested reactions such as like on comments or threaded comments. You can see this in action when you open the detail screen ;)
If you are using the iOS simulator to run the examples, make sure to use the Software Keyboard (⌘K)
Status Updates
Confused about "Status Updates"?
Let us know how we can improve our documentation:
Now that we have a working timeline with likes and comments, let’s add a status update screen to post content ourselves.
We will use TextToolBar for the input of the text. Add an instance variable textToolBar in our ViewController:
Let’s setup textToolBar in a separate method setupTextToolBar and call at the end of viewDidLoad (Make sure to add that after super.viewDidLoad())
Add this at the bottom of viewDidLoad() to show the status update form:
Our timeline view comes with refresh control included; if you scroll down the timeline you will get the new activity loaded.
Our timeline now has support for sharing status updates, the status update box comes with built-in support for:
- URL Previews
- Image Uploads
- Emoji and user mentions
Realtime Updates
Confused about "Realtime Updates"?
Let us know how we can improve our documentation:
As a final touch, we are connecting the timeline to Stream's real time APIs so that we can show a message each time a new activity is added to the feed.
To get FlatFeedViewController to listen for updates, we only need to call subscribeForUpdates(), that will create a Websocket connection to Stream APIs and subscribe for changes on the current feed.
Our feed now will show a notification every time a new activity is added. You can see this in action if you add a new activity.
Server-side Integration
Confused about "Server-side Integration"?
Let us know how we can improve our documentation:
So far we looked at how you can read and post activities using this sdk. In most cases you will also need to perform server-side interactions such as creating follow relationships, adding activities or change user-data. All the functionality we looked at in this tutorial is exposed via Stream's REST API and can be used server-side.
This is how we can add an activity from server-side:
Final Thoughts
In this tutorial we saw how easy it is to use Stream API and the iOS library to add a fully featured timeline to an application.
Adding feeds to an app can take weeks or months, even if you're a iOS developer. Stream makes it easy and gives you the tools and the resources to improve user engagement within your app. Time to add a feed!
Give us Feedback!
Did you find this tutorial helpful in getting you up and running with iOS for adding feeds to your project? Either good or bad, we’re looking for your honest feedback so we can improve.