React Audio Room Tutorial
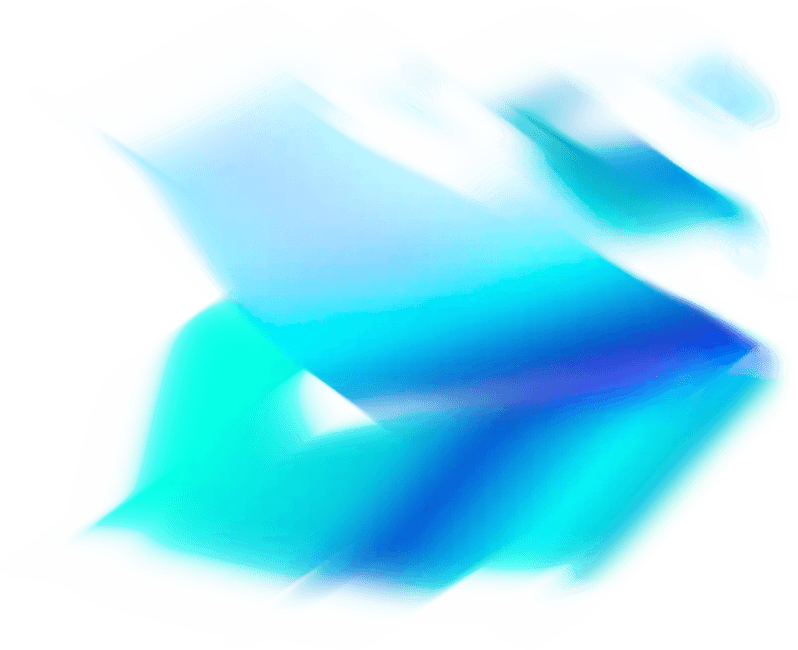
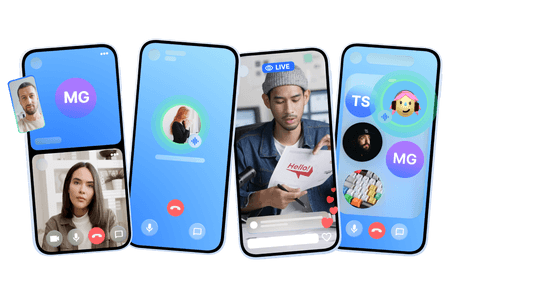
This tutorial will teach you how to build an audio room experience like Twitter Spaces or Clubhouse. The end result will look like the image below and will support the following features:
- Backstage mode. You can start the call with your co-hosts and chat a bit before going live
- Calls run on Stream's global edge network for optimal latency and scalability
- There is no cap to how many listeners you can have in a room
- Listeners can raise their hand, and be invited to speak by the host
- Audio tracks are sent multiple times for optimal reliability
Time to get started building an audio-room for your app.
Step 0 - Prepare your environment
Confused about "Step 0 - Prepare your environment"?
Let us know how we can improve our documentation:
For this tutorial, you'll need a few tools to be installed on your device. You can skip this step in case you already have them installed.
Step 1 - Create a new React app and install the React Video & Audio SDK
Confused about "Step 1 - Create a new React app and install the React Video & Audio SDK"?
Let us know how we can improve our documentation:
In this step, we will create a new React application using the Vite CLI, and install Stream's React Video & Audio SDK. We recommend using Vite because its fast and easy to use.
Step 2 - Create & Join a call
Confused about "Step 2 - Create & Join a call"?
Let us know how we can improve our documentation:
Open up src/App.tsx
and replace it with this code:
Let's review the example above and go over the details.
User setup
First we create a user object. You typically sync your users via a server side integration from your own backend. Alternatively, you can also use guest or anonymous users.
Client setup
Next, we initialize the client by passing the API Key, user and user token.
Create and join call
After the user and client are created, we create a call like this:
- This joins and creates a call with the type:
audio_room
and the specifiedcallId
- The users with id
john_smith
andjane_doe
are added as members to the call - And we set the
title
anddescription
custom field on the call object
In production grade apps, you'd typically store the call
instance in a state variable and take care of correctly disposing it.
Read more in our Joining and Creating Calls guide.
To actually run this sample we need a valid user token. The user token is typically generated by your server side API. When a user logs in to your app you return the user token that gives them access to the call. To make this tutorial easier to follow we'll generate a user token for you:
Please update REPLACE_WITH_USER_ID, REPLACE_WITH_TOKEN and REPLACE_WITH_CALL_ID with the actual values shown below:
Here are credentials to try out the app with:
Property | Value |
---|---|
API Key | Waiting for an API key ... |
Token | Token is generated ... |
User ID | Loading ... |
Call ID | Creating random call ID ... |
With valid credentials in place, we can join the call.
Step 3 - Adding audio room UI elements
Confused about "Step 3 - Adding audio room UI elements"?
Let us know how we can improve our documentation:
In this next step we'll add:
- Room title and description
- Controls to toggle live mode on/off
- A list of participants with their speaking status
Room Title & Description
Let's create the components we need to render this and add them to the main app
That's it for the basics, here's how the app MyUILayout
should look like now:
Finally, let's add the UILayout
to the main app component:
The approach is the same for all components.
We take the states of the call by observing call.state
updates through the SDK provided hooks,
such as useParticipants()
or useCallCustomData()
and use it to power our UI.
These two hooks and many others are available in the useCallStateHooks
utility.
In React, all call.state
properties can be accessed via a set of hooks, all exposed by the useCallStateHooks
utility hook.
This makes it easier to build UI components that react to changes in the call state.
Read more about it at Call & Participant State.
To make this a little more interactive, let's join the audio room from the browser.
Backstage & Live mode control
As you probably noticed by opening the same room from the browser, audio rooms by default are not live.
Regular users can only join an audio room when it is in live mode.
Let's expand the ControlPanel
and add a button that controls the backstage of the room.
While we're at it, let's also add a button that allows to mute/unmute the local audio track:
Now the app exposes a mic control button and a button that allows to toggle live mode on/off. If you try the web demo of the audio room you should be able to join as a regular user.
List Participants
As a next step, let's render the actual list of participants and show an indicator when they are speaking.
To do this we are going to create a Participant
component and render it from the ParticipantsPanel
With these changes things get more interesting, the app is now showing a list of all participants connected to the call and displays a green frame around the ones that are speaking.
However, you might have noticed that you can't hear the audio from the browser.
To enable this, you need to render the audio track of every participant.
Our SDK provides a special component for this purpose, called <ParticipantsAudio />
.
Let's add it to MyParticipantsPanel
:
Step 4 - Go live and join from the browser
Confused about "Step 4 - Go live and join from the browser"?
Let us know how we can improve our documentation:
If you now join the call from the browser, you will see that the participant list updates as you open/close the browser tab.
Note how the web interface won't allow you to share your audio/video.
The reason for this is that by default the audio_room
call type only allows moderators or admins to speak.
Regular participants can request permission.
And if different defaults make sense for your app you can edit the call type in the dashboard or create your own.
Step 4.1 - Enable Noise Cancellation
Confused about "Step 4.1 - Enable Noise Cancellation"?
Let us know how we can improve our documentation:
Background noise in an audio session is never a pleasant experience for the listeners and the speaker.
Our SDK provides a plugin that helps to greatly reduce the unwanted noise caught by your microphone. Read more on how to enable it here.
Step 5 - Requesting permission to speak
Confused about "Step 5 - Requesting permission to speak"?
Let us know how we can improve our documentation:
Requesting permission to speak is easy. Let's first have a quick look at how the SDK call object exposes this:
Requesting permission to speak
Handling permission requests
Permission requests are delivered to the call object in a form of an event one can subscribe to:
Let's add another view that shows the last incoming request as well as the buttons to grant / reject it
And here is the updated UILayout
code that includes it:
Step 6 - Group participants
Confused about "Step 6 - Group participants"?
Let us know how we can improve our documentation:
It is common for audio rooms and similar interactive audio/video experiences to show users in separate groups. Let's see how we can update this application to render participants in two separate sections: speakers and listeners.
Building custom layouts is very simple, all we need to do is to apply some filtering to the result of useParticipants()
hook.
We already have a view to display participants so all we need to do is to update it to use the new speakers
and listeners
arrays.
For simplicity, in this tutorial, we are skipping some of the best practices for building a production ready app. Take a look at our sample app linked at the end of this tutorial for a more complete example.
Other built-in features
Confused about "Other built-in features"?
Let us know how we can improve our documentation:
There are a few more exciting features that you can use to build audio rooms
- Query Calls: You can query calls to easily show upcoming calls, calls that recently finished as well as call previews.
- Reactions & Custom events: Reactions and custom events are supported.
- Recording & Broadcasting: You can record and broadcast your calls.
- Chat: Stream's Chat SDKs are fully featured and you can integrate them in the call
- Moderation: Moderation capabilities are built-in to the product
- Transcriptions: Transcriptions aren't available yet, but they are due to launch soon
Recap
Confused about "Recap"?
Let us know how we can improve our documentation:
It was fun to see just how quickly you can build an audio-room for your app. Please do let us know if you ran into any issues. Our team is also happy to review your UI designs and offer recommendations on how to achieve it with Stream.
To recap what we've learned:
- You set up a call with
const call = client.call('audio_room', '123')
- The call type
audio_room
controls which features are enabled and how permissions are set up - The
audio_room
by default enablesbackstage
mode, and only allows admins and the creator of the call to join before the call goes live - When you join a call, realtime communication is set up for audio:
await call.join()
- Call state
call.state
and utility state access hooks exposed throughuseCallStateHooks()
make it easy to build your own UI - For audio rooms, we use Opus RED and Opus DTX for optimal audio quality.
We've used Stream's Audio Rooms API, which means calls run on a global edge network of video servers. By being closer to your users the latency and reliability of calls are better. The React SDK enables you to build in-app video calling, audio rooms and livestreaming in days.
We hope you've enjoyed this tutorial and please do feel free to reach out if you have any suggestions or questions. You can find the code and the stylesheet for this tutorial in this CodeSandbox.
The source code for the companion audio room app, together with all of its features, is available on GitHub.
Final Thoughts
In this video app tutorial we built a fully functioning React messaging app with our React SDK component library. We also showed how easy it is to customize the behavior and the style of the React video app components with minimal code changes.
Both the video SDK for React and the API have plenty more features available to support more advanced use-cases.
Give us Feedback!
Did you find this tutorial helpful in getting you up and running with React for adding video to your project? Either good or bad, we’re looking for your honest feedback so we can improve.