You can have a look at the final result of this tutorial in the main
branch of the accompanying GitHub repository, and a packaged version of the game with a few additional features in the latest release of the Stream Chat Unreal Plugin.
Prerequisites
Before you get started, make sure you've installed the most recent version of Unreal Engine via the Epic Games launcher. You'll want to ensure you've selected "Templates and Feature Packs" when installing Unreal Engine (it is selected by default).
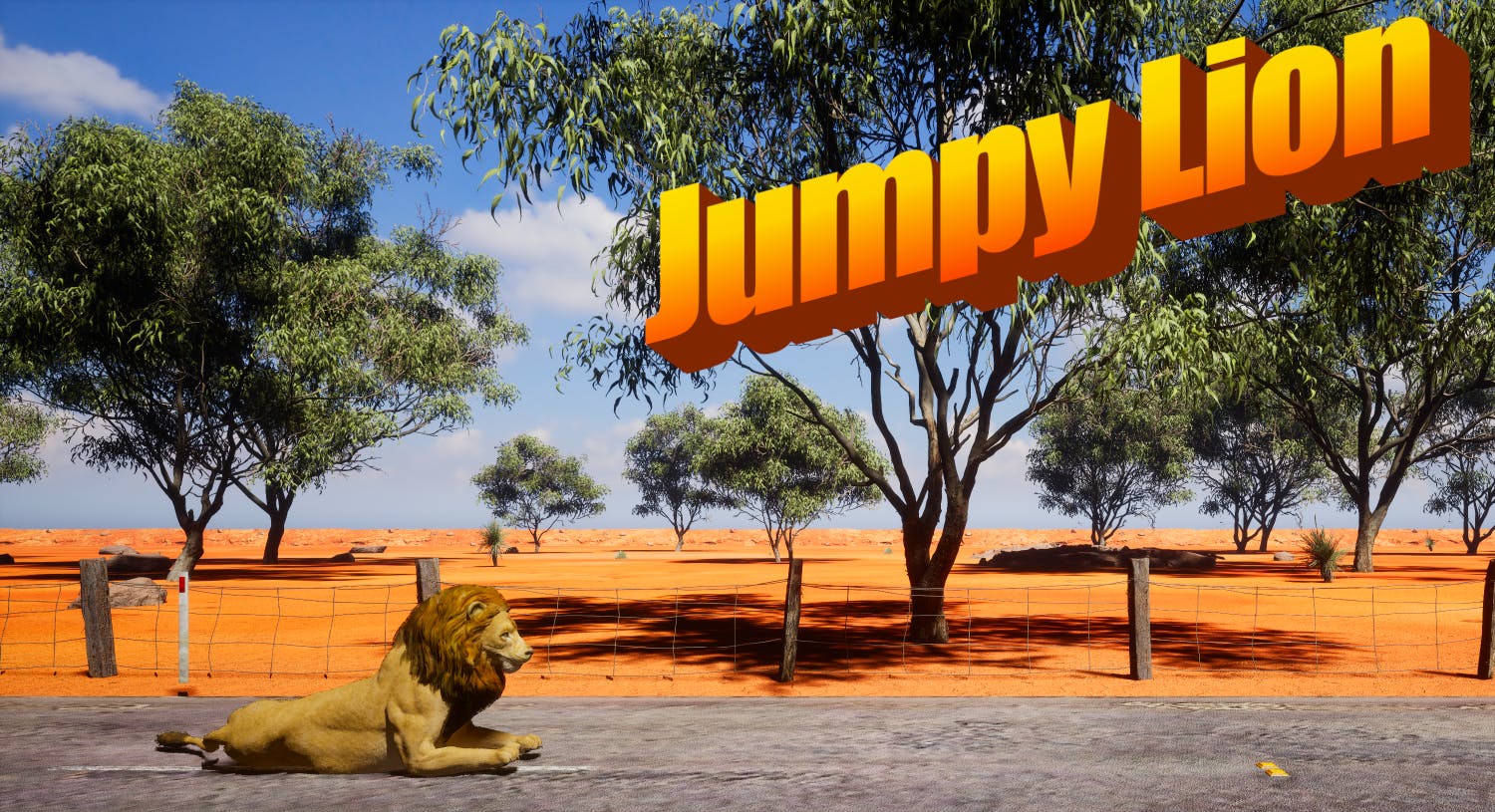
We’ll assume that you already have a game to which you want to add chat. For the purposes of this tutorial, we’ve quickly whipped up an immersive adventure, Jumpy Lion, using free assets available from the UE Marketplace.
If you’d like to follow along with this tutorial, clone the accompanying GitHub repository and checkout the start
branch.
How to Sign Up for Stream
Setting up a Stream Chat account is simple — head over to the Stream website and register with your GitHub account or with your email address.
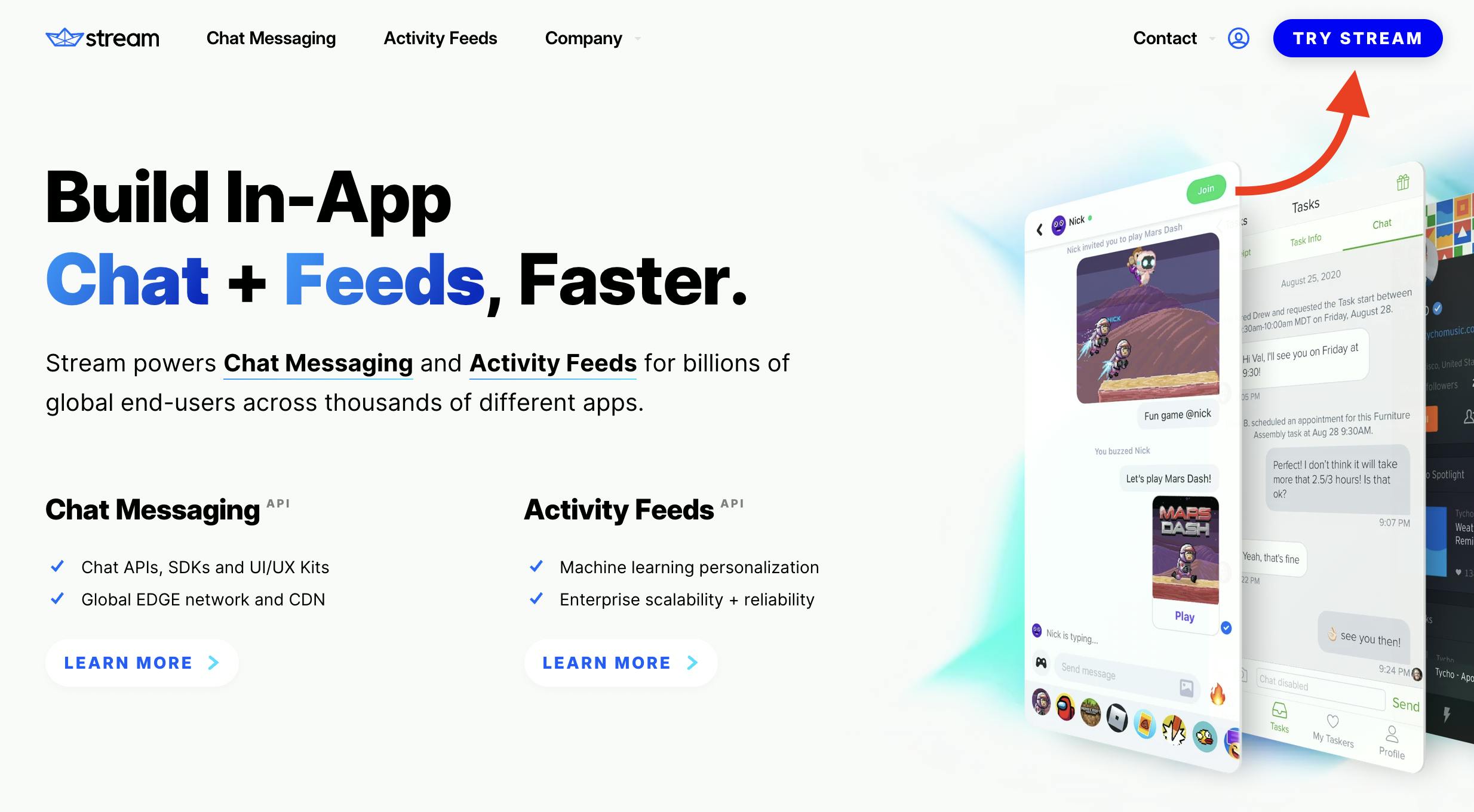
After registering, head over to the dashboard to find your API_KEY, as shown in the image below:

Keep this information in a secure place; you’ll need it to authenticate with the Stream API shortly.
You should also disable auth checks, as this will simplify the login process:

Download and Enable the Stream Chat Unreal Plugin
The Unreal Chat SDK includes the core functionality you need to interact with the Stream Game Chat API, with support for messages, channels, reactions and more. The SDK also incorporates a selection of Widgets to get you started building your own chat user experience in Unreal.
First, make sure you've downloaded the latest release of the Stream Chat plugin from the Releases page of the GitHub repository and copied it to the Plugins directory of your project.
Next, enable the Stream Chat plugin in the Plugins panel.

Add the Stream Chat Client Actor Component
Next, open the /JumpyLion/Core/BP_GameState_JumpyLion
Blueprint and add a “Stream Chat Client” component. Set the API Key property of the new component to the API key you saved earlier.
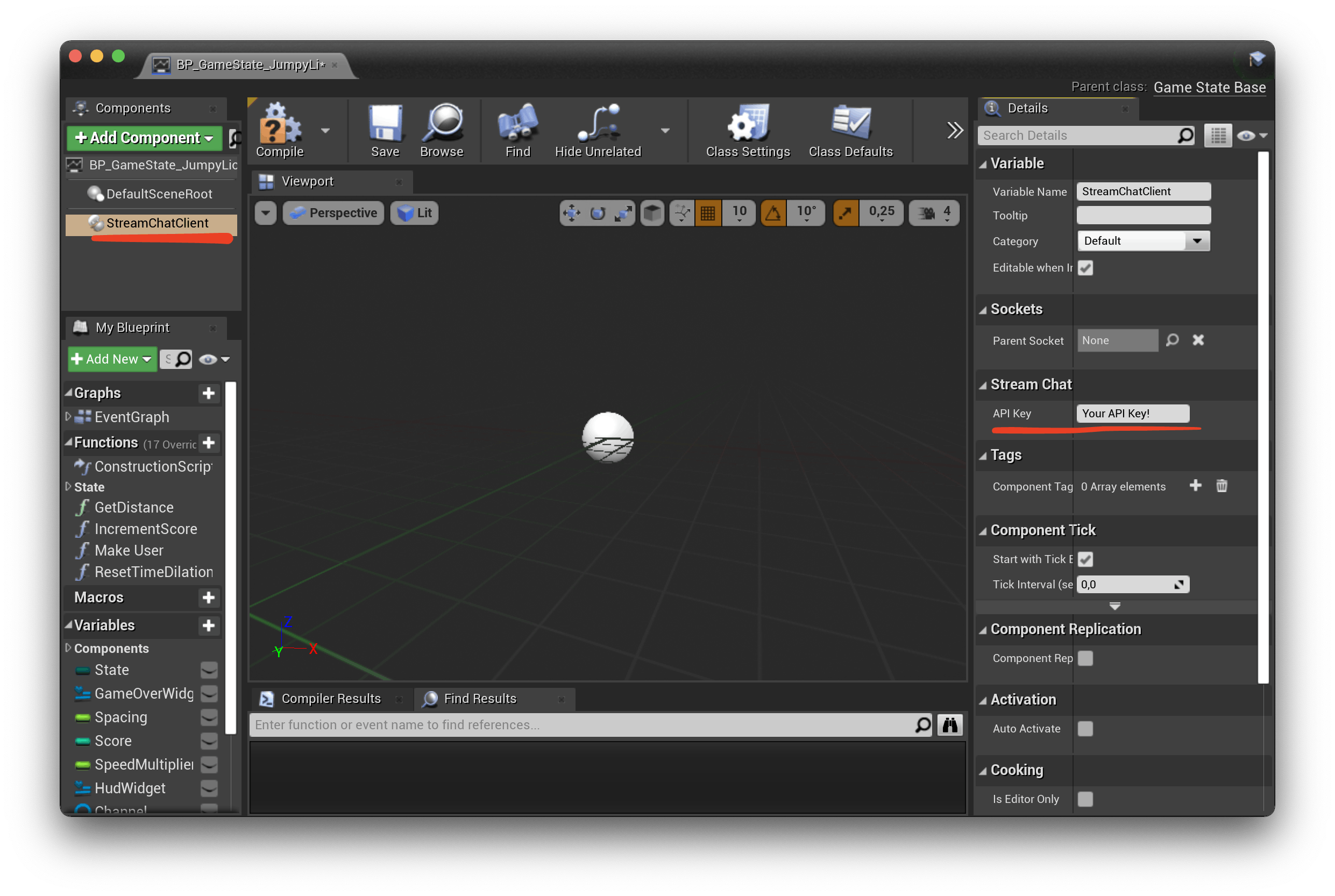
Connect User
The first thing you will always do when using Stream Chat is connect a user. To do this, use the “Connect User” Blueprint function available on the Stream Chat Client component. Create the following highlighted nodes in the Event Graph of the BP_GameState_JumpyLion
Blueprint, and hook them up to the BeginPlay event:
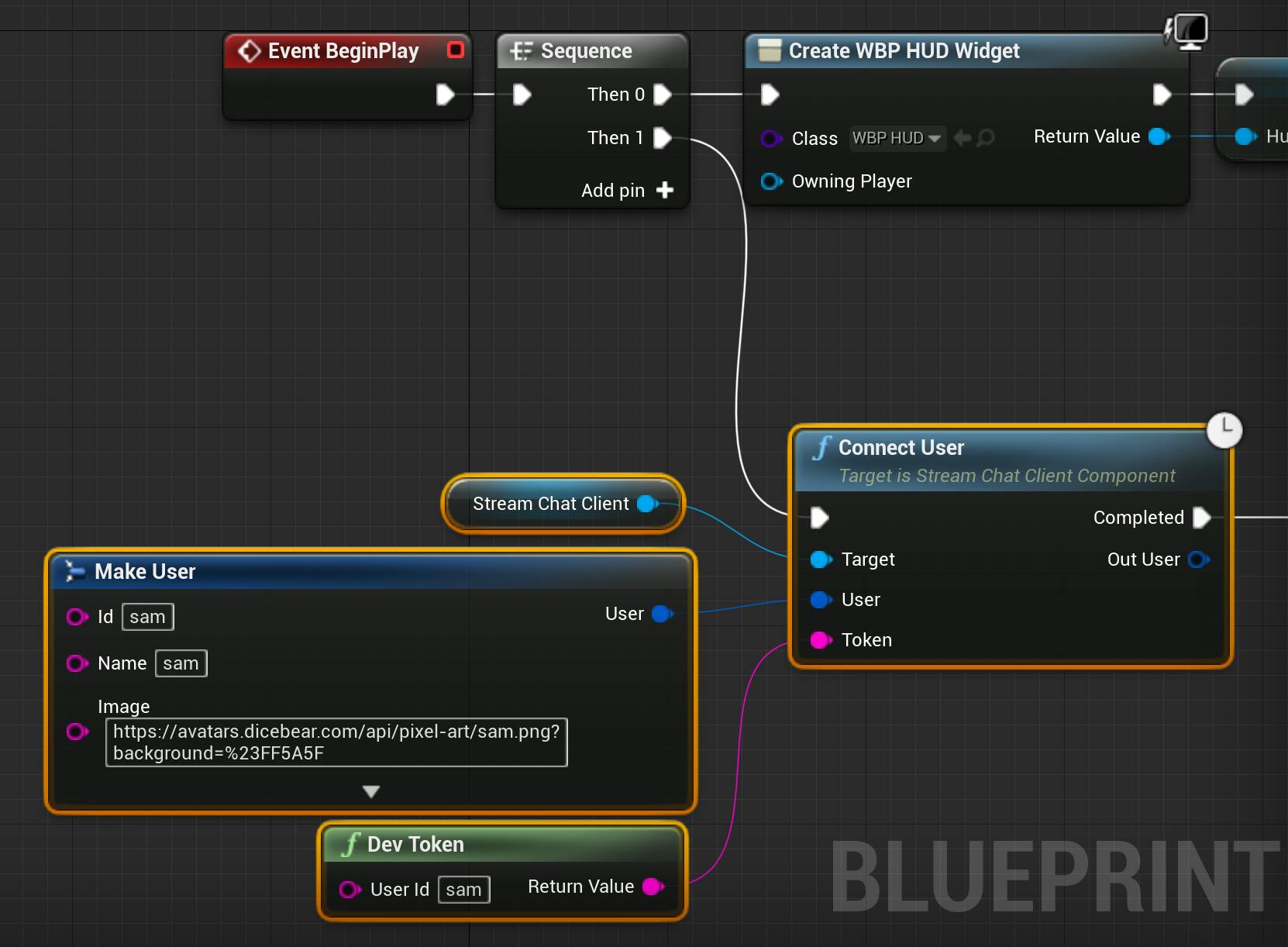
You can replace “sam” with anything you like, including in the image URL. As you disabled auth checks earlier, a token generated via the Dev Token node is sufficient to connect.
As this is a latent Blueprint node, the “Completed” pin will fire once you have successfully established a connection to the Stream Chat API.
Watch Channel
Next, we want to connect to a chat channel (creating it if necessary) and monitor it for new events. You can achieve this using the “Watch Channel” function, again on the Stream Chat Client component. Create the following nodes:

We’ve chosen to use the livestream
channel type, as it’s the most appropriate for a global chat channel. You can learn more about the different channel types in the Stream Chat docs.
We’ve also set an id
for the channel as well as a custom name
field using the Extra Data property. You can attach any kind of custom data to a channel; the name
field is very common for a human-readable description of the channel.
The “Watch Channel” node will return a ChatChannel
object once a response is successfully received from the Stream Chat API. As we want to reference this channel again later, we want to save a reference to this channel using a Blueprint variable.
Call the “Setup Chat” function on the HUD Widget. You’ll add functionality to this function in the next step.
Disconnect and move the existing “Set State” node and connect it after “Setup Chat”. This node enables the internal “Game” state, which begins the Jumpy Lion gameplay. Previously, we were happy for the game to start instantly. Now, we’ll wait for connection to the Stream Chat API before kicking off gameplay.

Add Chat Widget to HUD
Next, we’ll jump over to the /JumpyLion/UI/WBP_HUD
Widget Blueprint. Edit the existing “Setup Chat” function and add the following nodes:
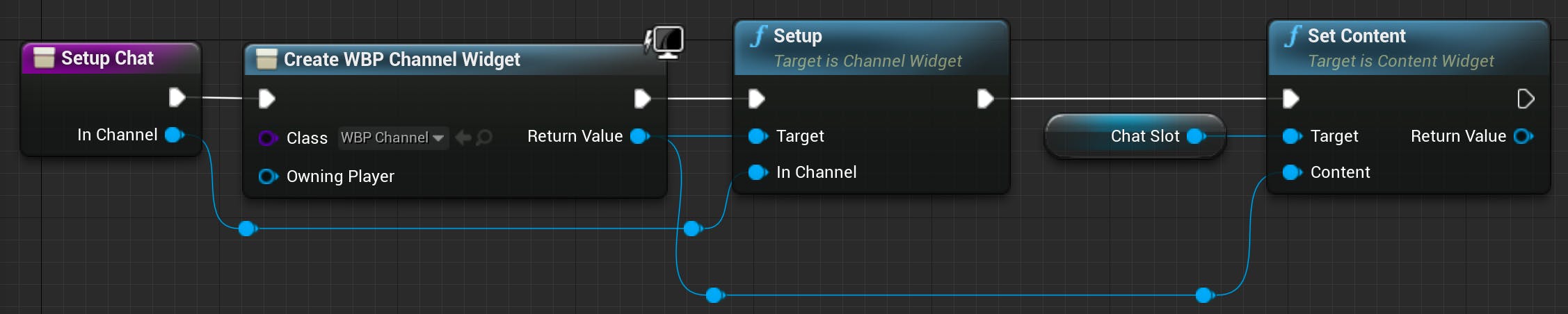
This simply creates a new instance of a WBP_Channel
widget, calls its “Setup” function, and adds the widget as the content of the “Chat Slot” named slot.
If you try to play your game in editor, you should already see the chat panel appear to the right of the screen. Once you lose the game, the mouse will appear and you will be able to type your own messages in the global chat.
We now have a fully-functional persistent chat integrated in our game, including things like messaging, reactions and context menu actions!

Customize the Chat Experience
We could end the guide here, but let’s explore the ways you can customize the chat experience for our use-case. In this game, every time a player loses the game, we want their high score to be posted in the chat. We also want this high score message to have a custom style, compared to the normal messages.
Let’s start with the simplest part, sending a message on game over. Edit the “Game Over State” function in “BP_GameState_JumpyLion” and add the following nodes after the final “Game Over” node:

First, check if the score is greater than zero - there’s no point in posting scores of zero to the chat! Next, use the “Send Message” function of the Chat Channel Component to send a message. Create some message text using the score value, and also add a custom high_score
field to the message and populate it with the current score value.
Now, if you test the game, every time you finish a run with a score greater than zero, a message will automatically appear in the chat!
Create a Global Chat Widget
We’d also like these messages to appear with a custom style. The default header panel of the WBP_Channel widget is also unnecessary for our purposes. Fix this by creating a custom chat Widget Blueprint, WBP_GlobalChat
in the UI directory.
Set Up the Widget Visuals
In the Designer view of the newly-created widget, you can remove the default Canvas widget in the widget tree and add a “Channel Context Widget” at the root.
The Channel Context widget allows any children in the widget tree to have access to the supplied
ChatChannel
object.
Next, add a “Vertical Box” as a child of the Channel Context Widget, and a “WBP_MessageList” and a “WBP_MessageComposer” as children of that, as shown below:

The Message Composer widget includes writing, sending, and editing functionality. The Message List widget will list all messages in a channel and includes functionality to paginate more messages when scrolling upwards in the chat.
Set the message list widget to take up all available space in the vertical box, by selecting it in the widget tree, and setting its slot’s Size in the Details panel to “Fill”:
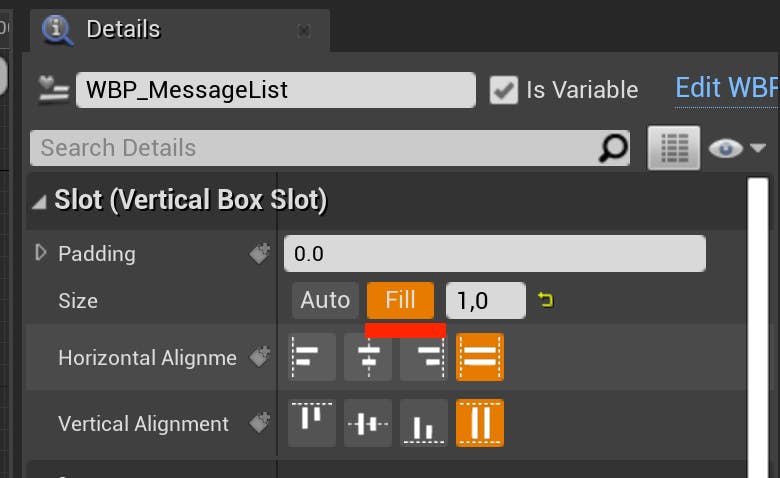
Script the Widget
Now, we want to customize the type of widget created when a new message arrives. To do this, we can override the “On Get Message” event of our Message List widget instance. Select the Message List instance in the widget tree, and create a binding on the “On Get Message Widget” event, as shown below:
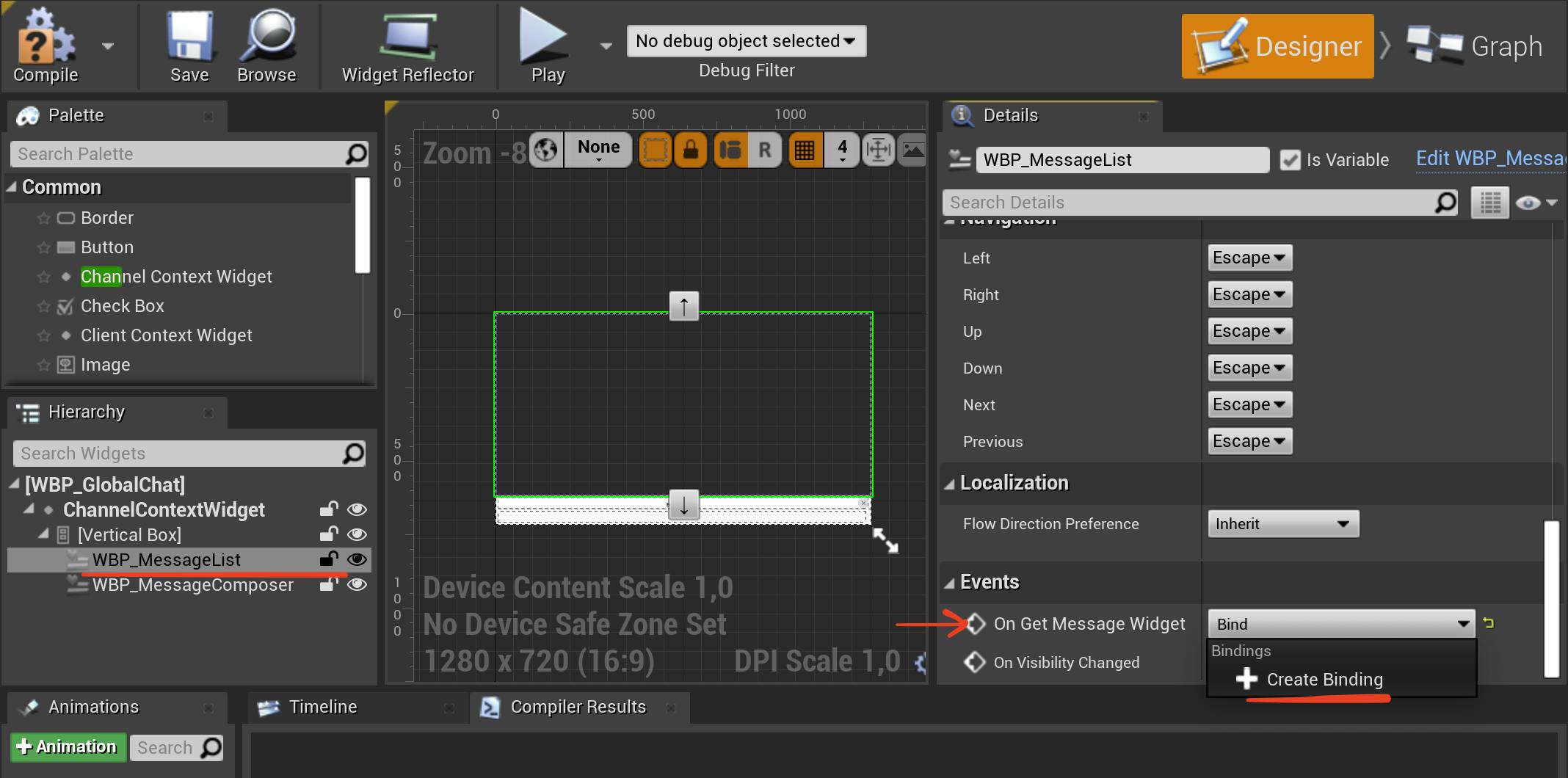
This will create a new function in the Graph tab of the “WBP_GlobalChat'' Widget Blueprint. The Message List widget calls this function for every message and expects an instance of a widget to be returned. We want to create a special widget if the message contains the high_score
field and otherwise the default widget. This is easily achieved with the following nodes:

Here, we’re using the “WBP_HighScoreMessage” widget for our custom widget. This widget has been set up with a different visual design and should already be included in the sample project. Feel free to have a look at its implementation.
Use the Global Chat Widget
The very last step is to use your newly-created global chat widget instead of the default team chat widget. Head back over to the WBP_HUD widget, edit the “Setup Chat” function to use the “WBP_GlobalChat” asset in the “Create Widget” node, as shown below:

Launch the game again, and you’ll see that all high scores in the message list are now shown with a custom design!

Conclusion
Don’t forget to check out the accompanying GitHub repository, which contains the final version of this project. You can also look at the implementation of the Jumpy Lion game, which is part of the main Unreal SDK repository. This version includes more features like a leaderboard and will continue to be updated in the future.