Talking to a therapist shouldn't feel like talking to tech support.
In this tutorial from Simon, you'll build a React Native mental health app that supports real-time chat, video consultations, and role-based access control. Clients and therapists can communicate through secure messaging and video calls, while therapists gain additional permissions to manage users and review session recordings.
You'll use Stream's Chat and Video SDKs to power the core communication features. The app uses React Native, Expo Router for navigation, and NativeWind for styling. A lightweight Node.js API handles authentication and generates secure user tokens for Stream.
The same architecture can support verticals like telehealth, edtech, finance, and other industries that require private, real-time communication.
Follow the tutorial to:
- Build a Node.js API to handle authentication and generate JWT (JSON Web Token)-based user tokens.
- Configure Stream roles and permissions for therapists and clients.
- Scaffold a React Native app using Expo and NativeWind.
- Create authentication screens with React Hook Form and Zod for form handling and validation.
- Integrate Stream Chat SDK for messaging, threads, reactions, and user management.
- Implement role-based navigation to control access for authenticated users.
- Use Stream Video SDK to enable secure video consultations.
- Record and transcribe video calls, and display recordings and transcripts in a therapist dashboard.
Overview of the Build
Set Up a Node.js API for Authentication
The tutorial starts by creating a Node.js API to issue Stream user tokens. This API authenticates users with JWT and exposes client and therapist registration endpoints. By handling token generation from the server side, you keep Stream credentials secure.
Configure Roles and Permissions in Stream
You'll define a custom therapist role in Stream's dashboard and assign permissions for creating channels, managing members, and moderating conversations. This allows therapists to access administrative features without exposing those capabilities to clients.
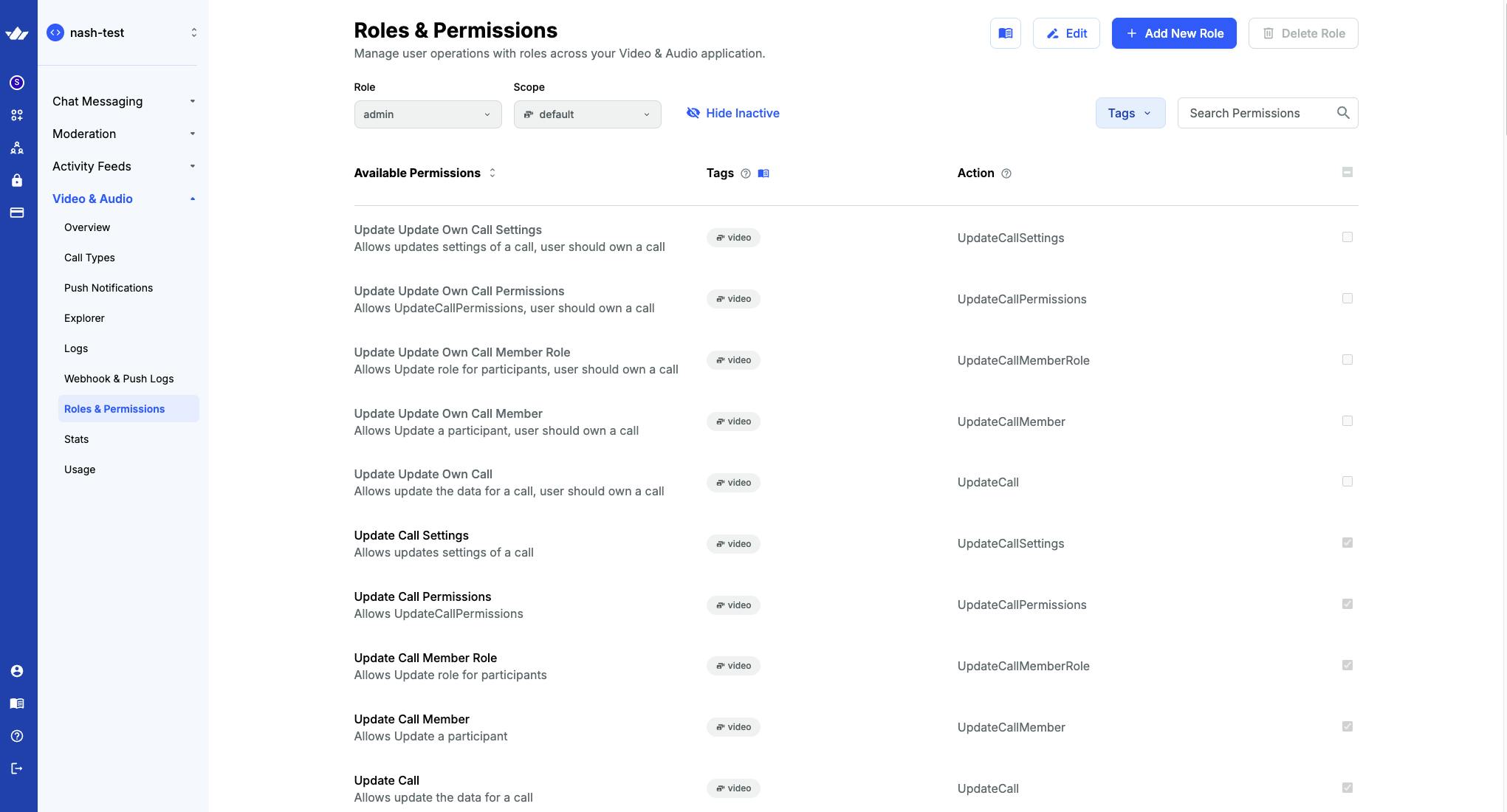
Scaffold the React Native App With Expo and NativeWind
The app is initialized with Expo Router for navigation and NativeWind for styling. You'll configure Metro and Babel settings to support Tailwind CSS and set up global styling for a consistent UI.
Build Authentication Screens With React Hook Form and Zod
The login and registration screens use React Hook Form for state management and Zod for validation. These screens interact with the Node.js API to authenticate users and store tokens securely with Expo SecureStore.
Integrate Stream Chat SDK
You'll add Stream Chat SDK to support real-time messaging. The tutorial covers creating chat channels, inviting members, and displaying a chat list with Stream's prebuilt UI components. You'll also implement threaded conversations and role-based UI behavior.
Implement Role-Based Navigation
The app uses Expo Router's folder-based routing to organize public and authenticated routes. Therapists and clients see different UI based on their role. Therapist-only features, like managing members and viewing session recordings, are restricted from client accounts.
Integrate Stream Video SDK
The tutorial integrates Stream Video SDK to enable live video consultations. Therapists can start a video call directly from a chat channel. The SDK handles low-latency streaming and connects authenticated users securely.
Display Recordings and Transcripts
Stream automatically generates session recordings and transcriptions. Therapists can access a dashboard view to review video recordings and transcripts linked to each consultation. This feature shows how to query Stream for media data and connect it to the app state.
What's Next?
Congratulations! You've built a feature-complete mental health app prototype with:
- 🔑 Role-based authentication
- 💬 Real-time chat and threaded messaging
- 🎥 Video consultations with recording and transcription
- 🗂️ Therapist-specific views for managing sessions and clients
Want to explore more features? Try adding:
Check out Simon's full video tutorial to follow along step-by-step, dive deeper into the code, and extend this project for your use case.