Stream now provides a fully-featured Jetpack Compose Chat SDK that you can use instead of the basic approach described in this article. Check out the Compose Chat Messaging Tutorial and give it a try today!
Intro and context
In our previous article about Jetpack Compose, we shared our initial impressions and recommended some learning resources. This time, we'll take Compose out for a spin by building a chat UI as an example implimentation with it!
Of course, you might be wondering why we're building a Compose example app when there's an official Google sample, Jetchat, which is also a chat app using Compose. That sample is a basic UI demo with a small bit of hardcoded data. Here, we're building a real, functional chat app instead that's hooked up to a real-time backend service.
We already ship a UI Components library in our Chat SDK which contains ready-to-go, fully-featured Android Views to drop into your app, along with ViewModels that let you connect them to business logic in just a single line of code. You can check out how that works in our Android Chat Tutorial.

This time, we're going to reuse the ViewModels (and a View) from that implementation, and build our Compose-based UI on top of that. This won't be an ideal scenario, as those ViewModels are designed to work with the Views that they ship with.
Having dedicated support for Compose in the SDK would make this much nicer and simpler, and that's something we'll be working on in the future. However, as you'll see, it's already quite easy to integrate Stream Chat with Jetpack Compose, without any specific support for it!
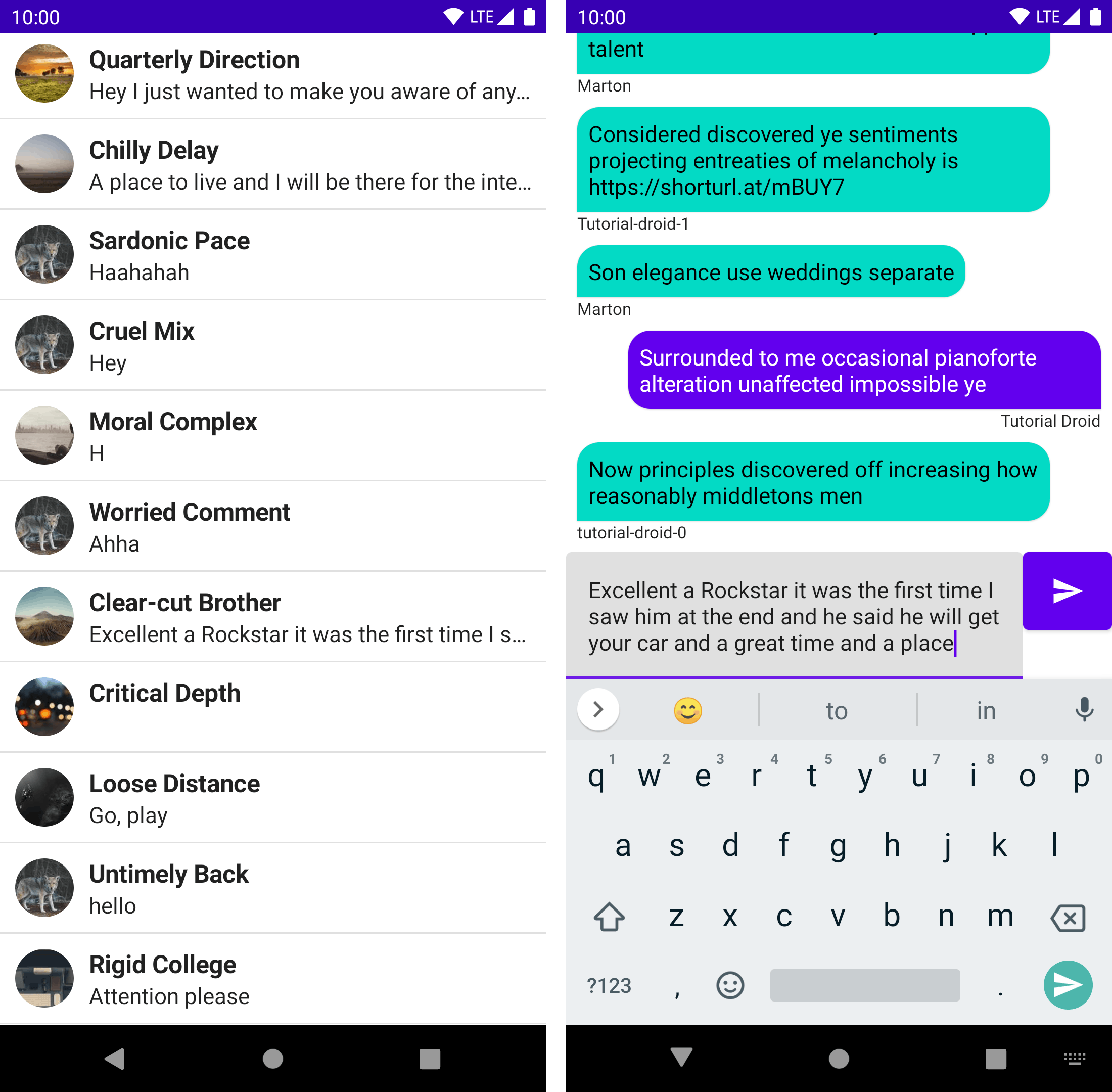
The project we're building in this article is available on GitHub, feel free to clone the project and play around with it on your own! We recommend getting your own Stream API key for this, which you can do by signing up for a free trial. Stream is free for hobby projects and small companies even beyond the trial - just apply for a free Maker account.
Project setup
To use Jetpack Compose, we'll create our project in the latest Canary version of Android Studio. This contains a project template for Compose apps.
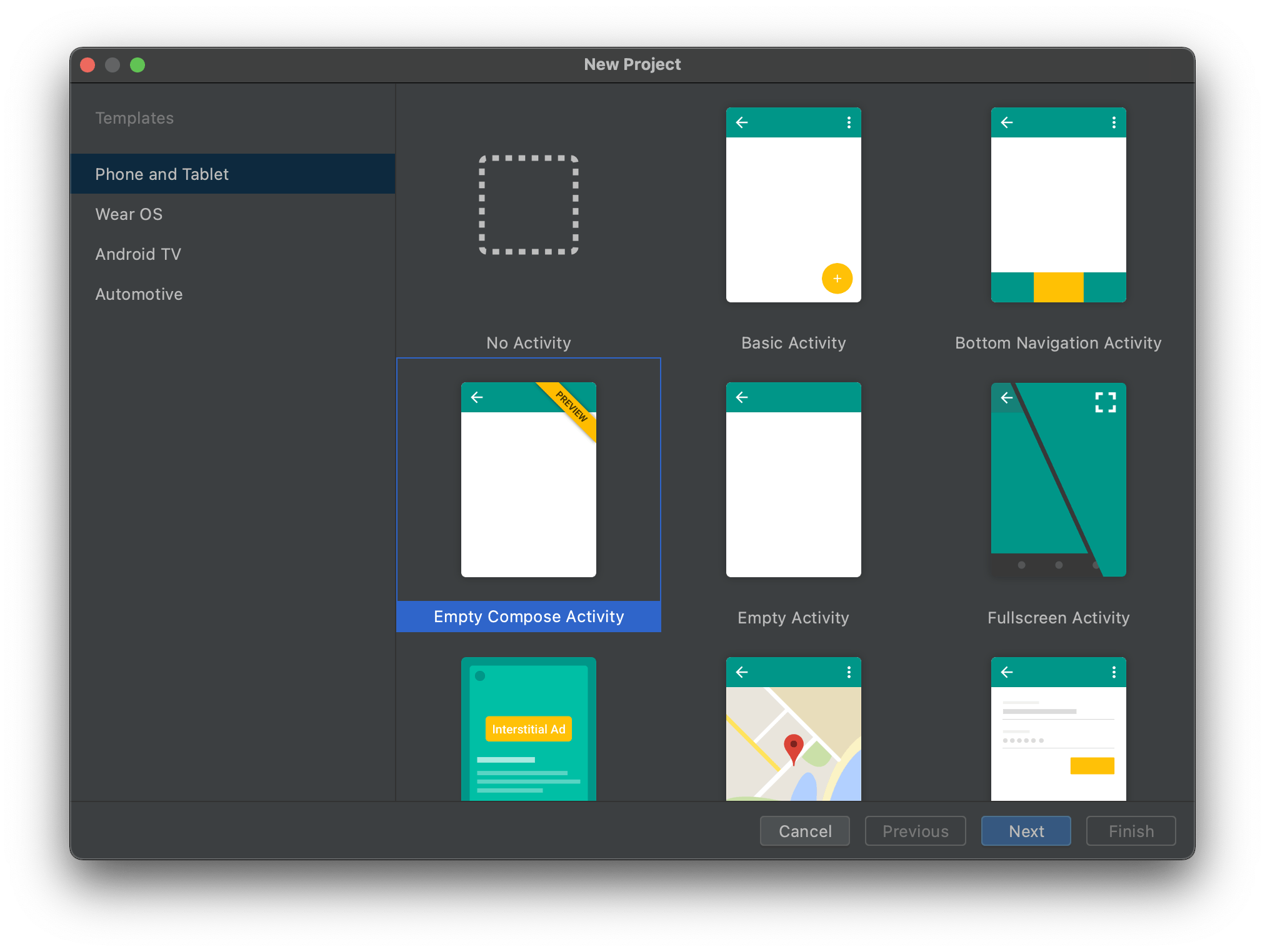
After creating the project, our first step will be to add the Stream UI Components SDK as a dependency, as well as some Jetpack Compose dependencies we need. Some of our dependencies come from Jitpack, so make sure to add that repository in the settings.gradle
file:
123456dependencyResolutionManagement { repositories { ... maven {url "https://jitpack.io" } // Add Jitpack } }
Then, in the module's build.gradle
file, replace the dependencies
block with the following:
123456789101112131415161718dependencies { // Stream Chat UI Components implementation "io.getstream:stream-chat-android-ui-components:4.8.1" // Google / Jetpack dependencies implementation "androidx.compose.runtime:runtime:$compose_version" implementation "androidx.compose.runtime:runtime-livedata:$compose_version" implementation 'androidx.core:core-ktx:1.3.2' implementation 'androidx.appcompat:appcompat:1.2.0' implementation 'com.google.android.material:material:1.3.0' implementation "androidx.compose.ui:ui:$compose_version" implementation "androidx.compose.material:material:$compose_version" implementation "androidx.compose.ui:ui-tooling:$compose_version" implementation 'androidx.lifecycle:lifecycle-runtime-ktx:2.3.0' implementation "androidx.navigation:navigation-compose:1.0.0-alpha10" implementation 'androidx.activity:activity-compose:1.3.0-alpha06' implementation 'androidx.lifecycle:lifecycle-viewmodel-compose:1.0.0-alpha04' }
Check out the GitHub project if you get tangled up in the dependencies.
Next, we'll set up the Stream Chat SDK, providing an API key and user details to it. This is normally done in your custom Application
class. To make things simple, we'll use the same environment and user as our tutorial.
12345678910111213141516171819202122class ChatApplication : Application() { override fun onCreate() { super.onCreate() val client = ChatClient.Builder(appContext = this, apiKey = "b67pax5b2wdq").build() val user = User().apply { id = "tutorial-droid" image = "https://bit.ly/2TIt8NR" name = "Tutorial Droid" } ChatDomain.Builder(client, this) .offlineEnabled() .build() client.connectUser( user, "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VyX2lkIjoidHV0b3JpYWwtZHJvaWQifQ.NhEr0hP9W9nwqV7ZkdShxvi02C5PR7SJE7Cs4y7kyqg" ).enqueue() } }
We're initializing all three layers of our SDK here: the low-level client, the domain providing offline support, and the UI components. For more info about these, take a look at the documentation. Finally, we're connecting our user, using a hardcoded, non-expiring example token from the tutorial.
Since we created a custom Application
class, we have to update our Manifest to use this ChatApplication
when the app is launched. We'll also set windowSoftInputMode
on the MainActivity
so that we'll have better keyboard behaviour later on.
12345678910111213<?xml version="1.0" encoding="utf-8"?> <manifest ...> <application android:name=".ChatApplication" ...> <activity android:name=".MainActivity" ... android:windowSoftInputMode="adjustResize"> ... </activity> </application> </manifest>
Example Channel List Screen
First, we'll create a screen that lists the channels available to our user. The UI Components library contains a ChannelListView component for this purpose, and we can reuse its ViewModel here.
12345678910111213141516171819202122232425@Composable fun ChannelListScreen( channelListViewModel: ChannelListViewModel = viewModel( // 1 factory = ChannelListViewModelFactory() ), ) { val state by channelListViewModel.state.observeAsState() val channelState = state ?: return // 2 Box( // 3 modifier = Modifier.fillMaxSize(), contentAlignment = Alignment.Center, ) { if (channelState.isLoading) { CircularProgressIndicator() // 4 } else { LazyColumn(Modifier.fillMaxSize()) { // 5 items(channelState.channels) { channel -> ChannelListItem(channel) Divider() } } } } }
Let's see what this code does step-by-step:
- We use the
viewModel()
method provided by Compose to get a ViewModel for our current context. This allows us to specify a factory that creates a ViewModel, which we need in the case ofChannelListViewModel
to pass in some parameters to it. We'll stick to the default parameters of the SDK'sChannelListViewModelFactory
for now, which will filter for channels that our current user is a member of. - We observe the
LiveData
state in the ViewModel as a composableState
, and we copy it to a local variable so that smart casts will work on it correctly. We also skip rendering anything if thisState
happens to benull
. - We'll have the
ChannelListScreen
fill the entire screen, and center any content inside it. - If the state is loading, we'll display a basic progress indicator.
- Otherwise, we'll display the list of channels in a
LazyColumn
. Each item will be aChannelListItem
responsible for showing info for a single channel. We're also adding a simpleDivider
after each item.
Now, let's see how we can render a single ChannelListItem
in the list:
12345678910111213141516171819202122232425@Composable fun ChannelListItem(channel: Channel) { Row( // 1 modifier = Modifier .padding(horizontal = 8.dp, vertical = 8.dp) .fillMaxWidth(), verticalAlignment = Alignment.CenterVertically ) { Avatar(channel) // 2 Column(modifier = Modifier.padding(start = 8.dp)) { // 3 Text( text = channel.getDisplayName(LocalContext.current), style = TextStyle(fontWeight = FontWeight.Bold), fontSize = 18.sp, ) val lastMessageText = channel.messages.lastOrNull()?.text ?: "..." Text( text = lastMessageText, maxLines = 1, overflow = TextOverflow.Ellipsis, ) } } }
- We're rendering each item as a
Row
. - Inside that, we're adding an
Avatar
representing theChannel
at the start. - Then we add a
Column
, laying out two pieces ofText
vertically. These will contain the channel's title and a preview of the latest message. Note theLocalContext.current
API being used to grab aContext
in Compose, as well as theTextOverflow.Ellipsis
option that will make long messages behave nicely in the preview.
We have one last Composable to implement, the Avatar
used above. Our UI components SDK ships an AvatarView
with complex rendering logic inside it, which we don't want to reimplement for now. We can use Jetpack Compose's interop features to include our regular Android View
inside Compose UI.
This will be really simple, just a few lines of code to create a wrapper:
12345678@Composable fun Avatar(channel: Channel) { AndroidView( factory = { context -> AvatarView(context) }, update = { view -> view.setChannelData(channel) }, modifier = Modifier.size(48.dp) ) }
The AndroidView
function allows us to create... Well, an Android View
, which we get a Context
for. It also gives us a way to hook into Compose's state updates using its update
parameter, which will be executed whenever composable state (in our case, the Channel
object) changes. When that happens, we set the new Channel
on our AvatarView
.
Finally, we can add the ChannelListScreen
to MainActivity
:
123456789101112class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { ComposeChatTheme { Surface(color = MaterialTheme.colors.background) { ChannelListScreen() } } } } }
Building and running this code gives us a working list of channels loaded from Stream's backend, with performant scrolling thanks to LazyColumn
. Not bad for a hundred lines of code for the entire thing!
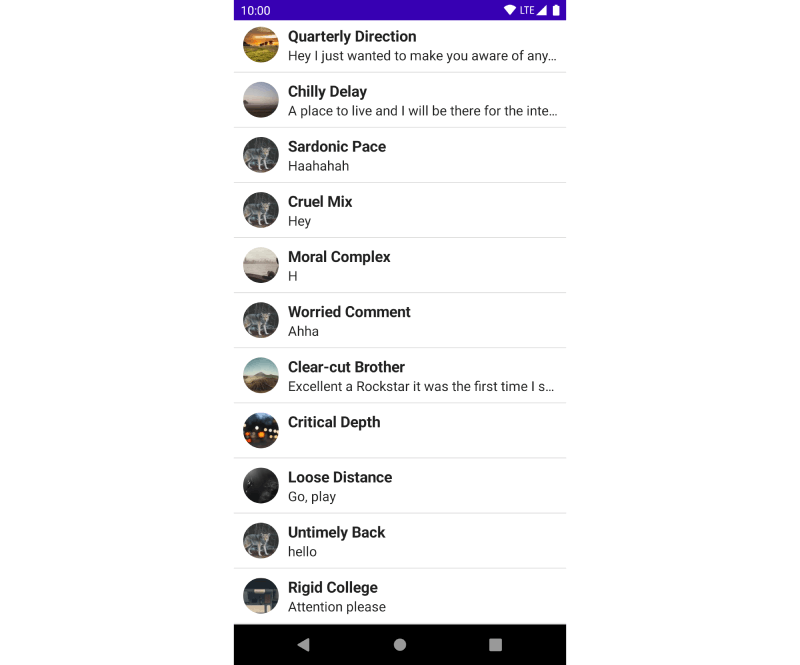
Setting up navigation
Continuing with our chat app, let's create a new screen, where we'll display the list of messages in a channel. For this, we'll need to set up click listeners and navigation. We'll use the Navigation Component for Jetpack Compose here.
Step one, we'll make our ChannelListItem
clickable, and add an onClick
parameter that it will call when it was clicked:
12345678910111213@Composable fun ChannelListItem( channel: Channel, onClick: (channel: Channel) -> Unit, // New callback parameter ) { Row( modifier = Modifier .clickable { onClick(channel) } // Add clickablity .padding(horizontal = 8.dp, vertical = 8.dp) .fillMaxWidth(), ... ) { ... } }
ChannelListScreen
will take a NavController
as its parameter, and use it to navigate to a new destination when an item was clicked, based on the cid
of the channel.
1234567891011121314151617@Composable fun ChannelListScreen( navController: NavController, // New parameter channelListViewModel: ChannelListViewModel = viewModel( factory = ChannelListViewModelFactory() ), ) { ... items(channelState.channels) { channel -> ChannelListItem( channel = channel, onClick = { navController.navigate("messagelist/${channel.cid}") }, ) Divider() } ... }
We'll update MainActivity
by moving our top-level Composable code to a ChatApp
composable, and setting up navigation in there.
12345678910111213141516171819202122232425262728class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { ComposeChatTheme { Surface(color = MaterialTheme.colors.background) { ChatApp() } } } } } @Composable fun ChatApp() { val navController = rememberNavController() // 1 NavHost(navController, startDestination = "channellist") { composable("channellist") { // 2 ChannelListScreen(navController = navController) } composable("messagelist/{cid}") { backStackEntry -> // 3 MessageListScreen( navController = navController, cid = backStackEntry.arguments?.getString("cid")!! ) } } }
- We're creating a
NavController
and aNavHost
for our application. - Our first and initial destination is a composable:
ChannelListScreen
. We pass the nav controller to it. - Our second destination will be a new composable called
MessageListScreen
. Here, we pass in both the nav controller and the argument that was used to navigate to it. We can grab this from theNavBackStackEntry
parameter.
Example Message List Screen
Our message list will be a LazyColumn
similar to the channel list we created before. For displaying messages, the UI Components library gives us a MessageListViewModelFactory
which can create a few different ViewModel instances. This takes the cid
of the channel we want to display.
1234567891011@Composable fun MessageListScreen( navController: NavController, cid: String, ) { val factory = MessageListViewModelFactory(cid) MessageList( navController = navController, factory = factory, ) }
We'll implement the MessageList
like this:
123456789101112131415161718192021222324252627282930313233343536373839@Composable fun MessageList( navController: NavController, factory: MessageListViewModelFactory, modifier: Modifier = Modifier, messageListViewModel: MessageListViewModel = viewModel(factory = factory), // 1 ) { val state by messageListViewModel.state.observeAsState() // 2 val messageState = state ?: return Box( modifier = modifier, contentAlignment = Alignment.Center ) { when (messageState) { // 3 is MessageListViewModel.State.Loading -> { CircularProgressIndicator() } is MessageListViewModel.State.NavigateUp -> { navController.popBackStack() } is MessageListViewModel.State.Result -> { val messageItems = messageState.messageListItem.items // 4 .filterIsInstance<MessageListItem.MessageItem>() .filter { it.message.text.isNotBlank() } .asReversed() LazyColumn( modifier = Modifier.fillMaxSize(), reverseLayout = true, // 5 ) { items(messageItems) { message -> MessageCard(message) // 6 } } } } } }
Let's review:
- We get a
MessageListViewModel
from the factory, which will fetch messages for us, and expose it asLiveData
. - As before, we convert the
LiveData
state from the ViewModel into composableState
. - We have three states to handle, defined by a sealed class inside
MessageListViewModel
. The first state is a loading state, the second is a state that pushes us to the previous screen (using the nav controller), and the third is the result state, where we have a list of messages. - In the result state, we'll grab the list of messages received, filter for only
MessageItem
s (excluding things such as date separators that we don't want to render for now). We also filter for messages that have non-blank text - for example, some messages might have only images attached to them, so we'll skip those for simplicity. Finally, we reverse the list, to match what we're doing in the next step. - We use
reverseLayout
onLazyColumn
to stack its items from the bottom. This is similar to usingstackFromEnd
on aRecyclerView
. - Each item will be rendered by a
MessageCard
composable.
For a single message, we'll use the following layout:
12345678910111213141516171819202122232425262728293031323334353637383940414243@Composable fun MessageCard(messageItem: MessageListItem.MessageItem) { // 1 Column( modifier = Modifier .fillMaxWidth() .padding(horizontal = 8.dp, vertical = 4.dp), horizontalAlignment = when { // 2 messageItem.isMine -> Alignment.End else -> Alignment.Start }, ) { Card( modifier = Modifier.widthIn(max = 340.dp), shape = cardShapeFor(messageItem), // 3 backgroundColor = when { messageItem.isMine -> MaterialTheme.colors.primary else -> MaterialTheme.colors.secondary }, ) { Text( modifier = Modifier.padding(8.dp), text = messageItem.message.text, color = when { messageItem.isMine -> MaterialTheme.colors.onPrimary else -> MaterialTheme.colors.onSecondary }, ) } Text( // 4 text = messageItem.message.user.name, fontSize = 12.sp, ) } } @Composable fun cardShapeFor(message: MessageListItem.MessageItem): Shape { val roundedCorners = RoundedCornerShape(16.dp) return when { message.isMine -> roundedCorners.copy(bottomEnd = CornerSize(0)) else -> roundedCorners.copy(bottomStart = CornerSize(0)) } }
This code is mostly straightforward, but let's review some of its important bits:
- We take a
MessageItem
as a parameter. - Depending on whether this is our current user's message, or someone else's, we align it to one of the sides of the screen. We also set colours based on this in a few places.
- We use a
cardShapeFor
helper method to create the shape of theCard
that holds the message. This will create a shape with rounded corners, except for one of the bottom corners, giving us a chat bubble look. - We display the username under each message, so that we can distinguish between messages sent by others.
At this point, we can build and run again, and clicking a channel will navigate to the new screen, displaying the list of messages in it.
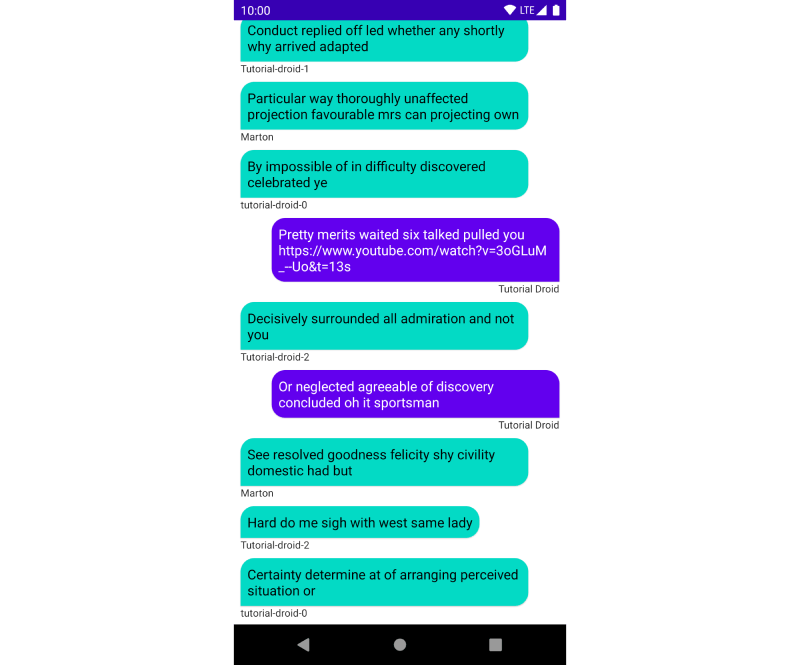
Message Input
For our final piece of Jetpack Compose chat implementation, we'll add an input view on the message list screen so that we can send new messages.
First, we'll modify MessageListScreen
and place a new composable under MessageList
:
12345678910111213141516@Composable fun MessageListScreen( navController: NavController, cid: String, ) { Column(Modifier.fillMaxSize()) { val factory = MessageListViewModelFactory(cid) MessageList( navController = navController, factory = factory, modifier = Modifier.weight(1f), ) MessageInput(factory = factory) } }
The weight
modifier on MessageList
will make it take up the maximum available space above the new MessageInput
. We pass in the factory
to MessageInput
as well, so that it's able to access the currently open channel's data via ViewModels.
1234567891011121314151617181920212223242526272829303132@Composable fun MessageInput( factory: MessageListViewModelFactory, messageInputViewModel: MessageInputViewModel = viewModel(factory = factory), // 1 ) { var inputValue by remember { mutableStateOf("") } // 2 fun sendMessage() { // 3 messageInputViewModel.sendMessage(inputValue) inputValue = "" } Row { TextField( // 4 modifier = Modifier.weight(1f), value = inputValue, onValueChange = { inputValue = it }, keyboardOptions = KeyboardOptions(imeAction = ImeAction.Send), keyboardActions = KeyboardActions { sendMessage() }, ) Button( // 5 modifier = Modifier.height(56.dp), onClick = { sendMessage() }, enabled = inputValue.isNotBlank(), ) { Icon( // 6 imageVector = Icons.Default.Send, contentDescription = stringResource(R.string.cd_button_send) ) } } }
- This time, we'll use a
MessageInputViewModel
, which normally belongs to the MessageInputView shipped in the UI components library, and handles input actions. - We create a piece of composable state to hold the current input value.
- This local helper function calls into the ViewModel and sends the message to the server. Then, it clears the input field by resetting the state.
- We use a
TextField
to capture user input. This displays the currentinputValue
, and modifies it based on keyboard input. We also set up IME options so that our software keyboard displays a Send button, and we handle that being tapped by callingsendMessage
. - We're also adding a
Button
that the user can tap to send a message. This is enabled/disabled dynamically based on the current input value. - The button will show an icon from the default Material icon set. Not to skimp on accessibility, we also add a content description string for the send icon. We grab this from regular Android resources using
stringResource
, which allows us to localize it as usual. Make sure to create this resource in your project.
Let's build and run for the last time, and we have a working chat app now! We can browse channels, open them, read messages, and send new messages.
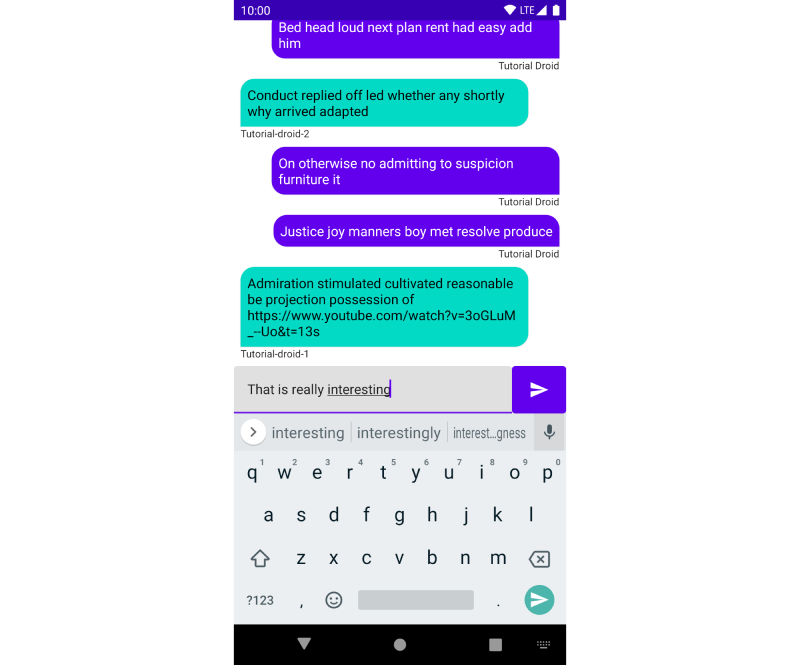
Conclusion
This complete implementation, including UI, logic, and a connection to a real server is roughly 250 lines of code in total. Almost all of this is UI code with Jetpack Compose, the integration with Stream Chat's ViewModels and Views is just a small fraction of it.
As a reminder, you can check out the full project and play with it on GitHub. We recommend expanding this example project to learn more about Compose - a good first goal could be to add a login screen, so that you can have different users on different devices.
To jump into that, sign up for a free trial of Stream Chat. If you're doing this for a side project or you're a small business, you can use our SDK for with a free Maker account, even beyond the trial period!
Here are some more useful links to continue exploring:
- Our comprehensive list of Jetpack Compose Tutorials for Beginners
- The Stream Chat Android SDK on GitHub, including the UI Components Sample app that showcases many features, and includes a login implementation you can take ideas from
- Our regular, non-Compose Android Tutorial
- The Stream Chat Android documentation
- For more about Compose, some first impressions and recommended learning resources