React Livestreaming Tutorial
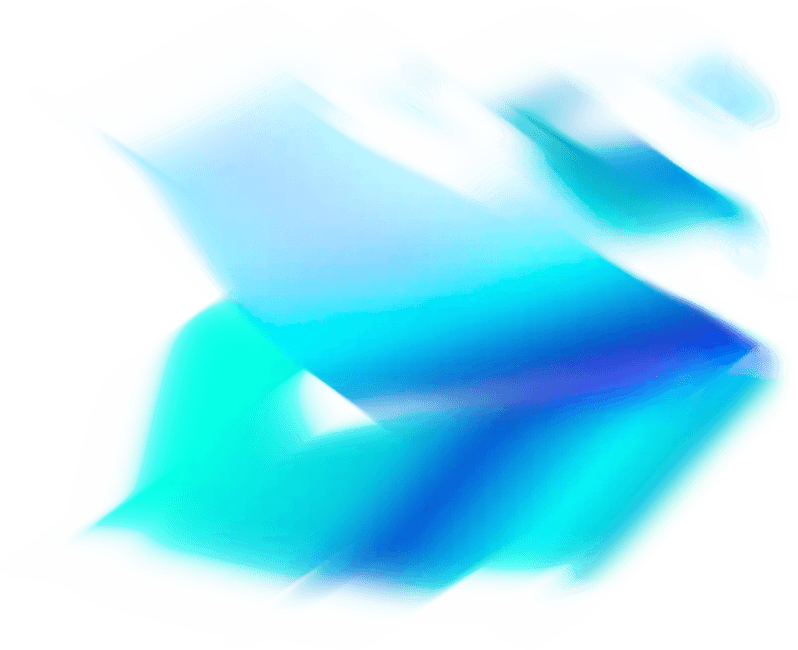
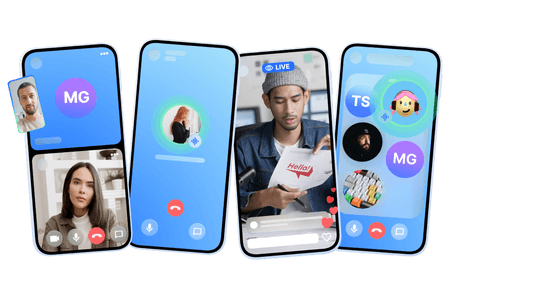
In this tutorial we'll quickly build a low-latency in-app livestreaming experience. The livestream is broadcasted using Stream's edge network of servers around the world.
We'll cover the following topics:
- Ultra low latency streaming
- Multiple streams & co-hosts
- RTMP in and WebRTC input
- Exporting to HLS
- Reactions, custom events and chat
- Recording & Transcriptions
Let's get started, if you have any questions or feedback be sure to let us know via the feedback button.
Step 0 - Prepare your environment
Confused about "Step 0 - Prepare your environment"?
Let us know how we can improve our documentation:
For this tutorial, you'll need a few tools to be installed on your device. You can skip this step in case you already have them installed.
Step 1 - Create a new React app and install the React Video SDK
Confused about "Step 1 - Create a new React app and install the React Video SDK"?
Let us know how we can improve our documentation:
In this step, we will create a new React application using the Vite CLI, and install Stream's React Video SDK. We recommend using Vite because its fast and easy to use.
Step 2 - Broadcast a livestream from your device
Confused about "Step 2 - Broadcast a livestream from your device"?
Let us know how we can improve our documentation:
The following code shows how to publish from your device's camera.
Let's open App.tsx
and replace its contents with the following code:
If you run the app now, you'll see that the client fails to connect. To fix that, we need to provide valid credentials. They shall be set in the following variables:
Replace them now with the appropriate values from the table below:
Here are credentials to try out the app with:
Property | Value |
---|---|
API Key | Waiting for an API key ... |
Token | Token is generated ... |
User ID | Loading ... |
Call ID | Creating random call ID ... |
When you run the app now you'll see a text message saying: TODO: render video
.
Before we get around to rendering the video let's review the code above.
User setup
First we create a user object. You typically sync these users via a server side integration from your own backend. Alternatively, you can also use guest or anonymous users.
Client setup
Next, we initialize the client by passing the API Key, user and user token.
Create and join call
The most important step to review is how we create the call. Stream uses the same call object for livestreaming, audio rooms and video calling. Have a look at the code snippet below:
To create the first call object, specify the call type as livestream
and provide a unique callId
.
The livestream call type comes with default settings that are usually suitable for livestreams, but you can customize features, permissions, and settings in the dashboard. Additionally, the dashboard allows you to create new call types as required. For more information, check the Call Types docs.
Finally, using call.join({ create: true })
will not only create the call object on our servers but also initiate the real-time transport for audio and video.
This allows for seamless and immediate engagement in the livestream.
You can also add members to a call and assign them different roles.
Also, in production grade apps, you'd typically store the call
instance in a state variable and take care of correctly disposing it.
Read more in our Joining and Creating Calls guide.
Step 2.1 - Enable Noise Cancellation
Confused about "Step 2.1 - Enable Noise Cancellation"?
Let us know how we can improve our documentation:
Background noise in a livestream is never a pleasant experience for the listeners and the hosts.
Our SDK provides a plugin that helps to greatly reduce the unwanted noise caught by your microphone. Read more on how to enable it here.
Step 3 - Rendering the video
Confused about "Step 3 - Rendering the video"?
Let us know how we can improve our documentation:
In this step we're going to build a UI for showing your local video with a button to start the livestream.
In App.tsx
replace the LivestreamUI
component with the following code:
Upon running your app, you will be greeted with an interface that looks like this:
Stream uses a technology called SFU cascading to replicate your livestream over different SFUs around the world. This makes it possible to reach a large audience in realtime.
Now let's press Start Livestream in your new app and click the Join Call button below to watch your livestream in another tab in your browser. Please, make sure the call is created using the credentials from section Step 2 before you click Join Call
:
Let's take a moment to review the code above.
You can see we use a few hooks to get the call state. The most important ones are:
The Call & Participant state lists all available hooks you can use for accessing call and participant state.
The layout is built using standard React DOM elements. The ParticipantView component is provided by Stream's React Video SDK. ParticipantView renders the video and a fallback. You can use it for rendering the local and remote video.
Backstage mode
In the example above you might have noticed the call.goLive()
method and the isCallLive
value.
The backstage functionality is enabled by default on the livestream call type.
It makes it easy to build a flow where you and your co-hosts can setup your camera and equipment before going live.
Only after you call call.goLive()
will regular users be allowed to join the livestream.
This is convenient for many livestreaming and audio-room use cases. If you want calls to start immediately when you join them that's also possible. Simply go the Stream dashboard, click the livestream call type and disable the backstage mode.
The call.goLive
method can also automatically start HLS livestreaming, recording or transcribing.
In order to do that, you need to pass the corresponding optional parameter:
Because of simplicity, in this tutorial, we are skipping some of the best practices for building a production ready app. Take a look at our sample app linked at the end of this tutorial for a more complete example.
Step 4 - (Optional) Publishing RTMP using OBS
Confused about "Step 4 - (Optional) Publishing RTMP using OBS"?
Let us know how we can improve our documentation:
The example above showed how to publish your device camera to the livestream. Almost all livestream software and hardware supports RTMPS. OBS is one of the most popular livestreaming software packages and we'll use it to explain how to import RTMPS. So let's see how to publish using RTMPs. Feel free to skip this step if you don't need to use RTMPs.
Log the URL & Stream Key
Open OBS and go to settings -> stream
- Select "custom" service
- Server: equal to the
rtmpURL
from the log - Stream key: equal to the
streamKey
from the log
Press start streaming in OBS. The RTMP stream will now show up in your call just like a regular video participant.
Now that we've learned to publish using WebRTC or RTMP let's talk about watching the livestream.
Step 5 - Viewing a livestream (WebRTC)
Confused about "Step 5 - Viewing a livestream (WebRTC)"?
Let us know how we can improve our documentation:
Watching a livestream is even easier than broadcasting.
Our SDK provides a built-in LivestreamLayout
that you can use out of the box. Read more about it here: Watching a livestream (WebRTC).
Keep in mind that this component respects the Call Type settings, and it may happen that audio and video are published automatically, unless explicitly disabled.
You can check the full example in this CodeSandbox.
Step 6 - Ending the livestream
Confused about "Step 6 - Ending the livestream"?
Let us know how we can improve our documentation:
There are two ways on how to end a livestream:
call.stopLive()
This operation will turn on backstage again, and the viewers will be kicked out of the call, but the hosts will remain in the call.
Similar like in step 3, call.goLive()
will mark the call open for viewers to join.
call.endCall()
This operation will end the call for all participants (including hosts). Unless the call type permissions are changed, neither the hosts nor the viewers will be able to join the same call instance again.
Step 7 - (Optional) Viewing a livestream with HLS
Confused about "Step 7 - (Optional) Viewing a livestream with HLS"?
Let us know how we can improve our documentation:
Another way to watch a livestream is using HLS. HLS tends to have a 10 to 20 seconds delay, while the above WebRTC approach is realtime. The benefit that HLS offers is better buffering under poor network conditions. So HLS can be a good option when:
- A 10-20 second delay is acceptable
- Your users want to watch the Stream in poor network conditions
One option to start HLS is to set the start_hls
parameter to true
in the call.goLive()
method, as described above.
If you want to explicitly start it, when you are live, you can use the following method:
You can view the HLS video feed using any HLS capable video player.
Step 8 - Advanced Features
Confused about "Step 8 - Advanced Features"?
Let us know how we can improve our documentation:
This tutorial covered broadcasting and watching a livestream. It also went into more details about HLS & RTMP-in.
There are several advanced features that can improve the livestreaming experience:
- Co-hosts You can add members to your livestream with elevated permissions. So you can have co-hosts, moderators etc.
- Custom events You can use custom events on the call to share any additional data. Think about showing the score for a game, or any other realtime use case.
- Reactions & Chat Users can react to the livestream, and you can add chat. This makes for a more engaging experience.
- Recording The call recording functionality allows you to record the call with various options and layouts
Recap
Confused about "Recap"?
Let us know how we can improve our documentation:
It was fun to see just how quickly you can build in-app low latency livestreaming. Please do let us know if you ran into any issues. Our team is also happy to review your UI designs and offer recommendations on how to achieve it with Stream.
To recap what we've learned:
- WebRTC is optimal for latency, HLS is slower but buffers better for users with poor connections
- You setup a call:
const call = client.call("livestream", callId)
- The call type
"livestream"
controls which features are enabled and how permissions are setup - The livestream by default enables "backstage" mode. This allows you and your co-hosts to setup your mic and camera before allowing people in
- When you join a call, realtime communication is setup for audio & video:
await call.join()
- Call state
call.state
and helper state access hooks exposed throughuseCallStateHooks
make it easy to build your own UI
We've used Stream's Livestream API, which means calls run on a global edge network of video servers. By being closer to your users the latency and reliability of calls are better. The React SDK enables you to build in-app video calling, audio rooms and livestreaming in days.
We hope you've enjoyed this tutorial and please do feel free to reach out if you have any suggestions or questions. You can find the code for this tutorial in this CodeSandbox.
The source code for the companion Livestreaming App, together with all of its features, is available on Github.
Final Thoughts
In this video app tutorial we built a fully functioning React messaging app with our React SDK component library. We also showed how easy it is to customize the behavior and the style of the React video app components with minimal code changes.
Both the video SDK for React and the API have plenty more features available to support more advanced use-cases.
Give us Feedback!
Did you find this tutorial helpful in getting you up and running with React for adding video to your project? Either good or bad, we’re looking for your honest feedback so we can improve.