With the advent and increasing popularity of artificial intelligence (AI) large language models (LLMs) like ChatGPT and Google's Gemini, many customers wish to implement these solutions to maintain feature parity in the marketplace and provide highly engaging and memorable experiences for their user base.
As an industry-leading solution, Stream evolves alongside these market shifts and provides an easy implementation with ChatGPT. Follow these simple steps to supercharge your app with ChatGPT with a lightning fast implementation.
First, set up an OpenAI account, create an API Key, head to the docs page, and navigate to the tutorial here for setting up your OpenAI API credentials locally.

Next, you will use your existing Stream Chat App or create a new Stream Chat App by clicking “Create App” in the dashboard.

To quickly test this implementation locally, you can then set up a local server to receive all of your API requests from the Stream webhook with Ngrok. Set up a Ngrok local server, create a free account, and then add an auth token.
Auth token command & Ngrok local server for testing.

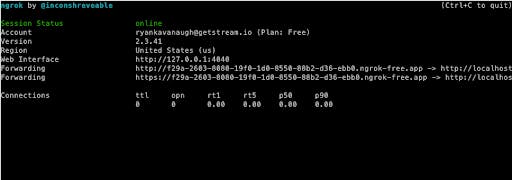
Next, set the Stream webhook to send events to your Ngrok Server by including this link in your Webhook URL below. For this example, we assume you set the Port the 80 for the Ngrok server.
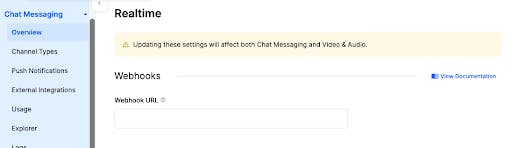
Now, we will create a NodeJS script that will exist server-side for your production deployment and create the 2-way communication between Stream Chat and ChatGPT. In this example, it will run locally on Port 80.
Receive the Stream new message webhook event.
123456789101112app.post("/", (req, res) => { let body=""; req.on("data", (chunk) => { body+=chunk; }); // Payload from Stream Webhook req.on("end", async () => { let parsedBody = JSON.parse(body); if(parsedBody.message==undefined){ return }
Next, save the message sent by the user, the Channel ID, Channel Type, and User ID.
12345// Capture all the relevant data from the Stream message object const channelID = parsedBody.channel.id const channelType = parsedBody.channel.type const incomingMessage = parsedBody.message.text.toLowerCase() const user = parsedBody.message.user.id
Now, we will send the user’s message to ChatGPT.
12345// Send Chat GPT the user's message const GPTResponse = await openai.chat.completions.create({ messages: [{ role: "system", content: incomingMessage }], model: "gpt-3.5-turbo", });
Finally, we will send the message response from ChatGPT back to the same channel and from a user named “ChatGPT, AI Assistant, etc.”
123456789101112131415// Separate out GPT's reponse from other data fields let messageResponse = GPTResponse.choices[0].message.content // Send the Chat GPT response back as a message to the same channel // Initialize Chat & fetch the channel const client = StreamChat.getInstance(process.env.KEY,process.env.SECRET); const channel = client.channel(channelType, channelID, {}) // Send GPT response to the channel const gptmessage = { text: messageResponse, user_id: AIAssitant }; channel.sendMessage(gptmessage); }
Here is the entire script for connecting Stream to ChatGPT.
123456789101112131415161718192021222324252627282930313233343536373839404142434445464748495051525354555657585960616263646566676869707172737475767778const cors = require("cors"); const port = process.env.PORT || 80; const express = require("express") const app = express(); const OpenAI = require("openai"); const openai = new OpenAI(); const StreamChat = require('stream-chat').StreamChat; require('dotenv').config() // ==================================================================== // This Chat GPT Implementation is created in 3 easy steps: // // Step 1: Save relevant data from the Stream message object provided via webhook event // Step 2: Send Chat GPT the user's message/question and get the response // Step 3. Send the Chat GPT reponse back as a message to the same channel // // Note: You can use the Stream frontend SDKs to easily render this conversation // for the end user with a modern, feature-rich, polished UI // ==================================================================== const AIAssitant = 'ChatGPTUserName' // Middleware app.use(cors()); // This is where all of the main code is executed app.post("/", (req, res) => { let body=""; req.on("data", (chunk) => { body+=chunk; }); // Payload from Stream Webhook req.on("end", async () => { let parsedBody = JSON.parse(body); if(parsedBody.message==undefined){ return } // Step 1. Capture relevant data from the Stream message object const channelID = parsedBody.channel.id const channelType = parsedBody.channel.type const incomingMessage = parsedBody.message.text.toLowerCase() const user = parsedBody.message.user.id // Step 2. Send Chat GPT the user's message/question and get the response try { // Confirming the message was sent by the user if (user != AIAssitant) { // Send Chat GPT the user's message const GPTResponse = await openai.chat.completions.create({ messages: [{ role: "system", content: incomingMessage }], model: "gpt-3.5-turbo", }); // Separate out GPT's reponse from other data fields let messageResponse = GPTResponse.choices[0].message.content // Step 3. Send the Chat GPT reponse back as a message to the same channel // Initialize Chat & fetch the channel const client = StreamChat.getInstance(process.env.KEY,process.env.SECRET); const channel = client.channel(channelType, channelID, {}) // Send GPT response to the channel const gptmessage = { text: messageResponse, user_id: AIAssitant }; channel.sendMessage(gptmessage); } } catch (error) { console.log(error); } res.status(200).send("OK"); }); }); app.listen(port, () => { console.log(`server running on port ${port}`); });
Now you have successfully implemented ChatGPT with Stream Chat! Please contact us with any questions.