Overview
Stream SDK aims to make it as easy as possible to build your own video calling, audio rooms, and live streams. We support a low-level client, guides on building your own UI, and several pre-built UI components. If you quickly want to add calling to your app, you can do that just in an hour with these UI components.
Rendering Participant
If you want to render a participant's video together with:
- A label/name for the participant
- Network quality indicator
- Mute/unmute indicator
- Fallback for when video is muted
- Reactions
We can use ParticipantView:
<ParticipantView participant={participant} />
You will see the result as below:


Video Call UI
You can use the CallContent
:
- Header: Content is shown that calls information or additional actions like back button, participant info.
- Call Participants Layout: A call video that renders the full participants of the call.
- Controls: Content is shown that allows users to trigger different actions to control a joined call.
const App = () => {
return (
<View style={styles.container}>
<CallContent />
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
},
});
Ringing (Incoming/Outgoing calls)
You can implement incoming/outgoing screens using our RingingCallContent
component:
- It displays the
IncomingCall
/OutgoingCall
components depending upon the call states. - After the call is accepted its displays the
CallContent
component. - While the call is in joining state it shows
JoiningCallIndicator
component.
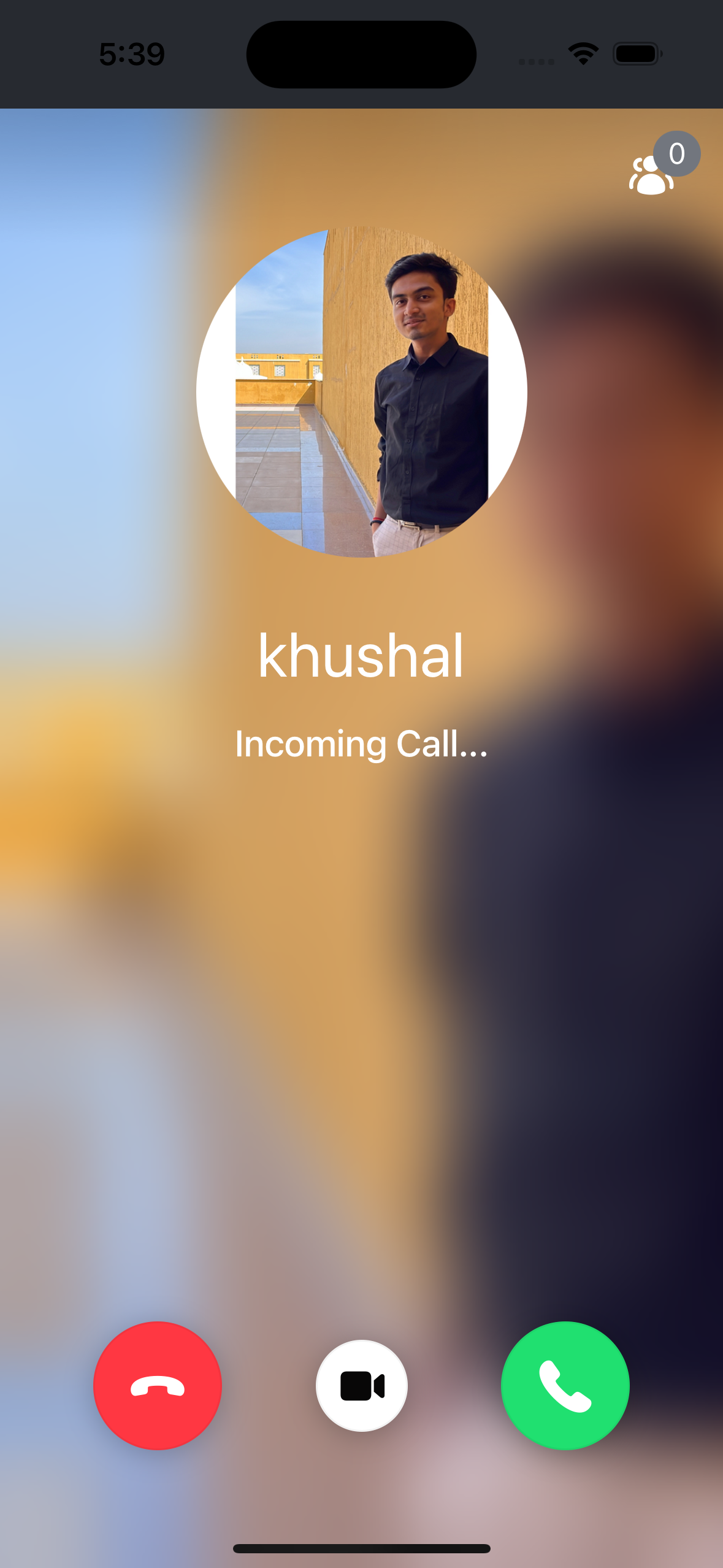
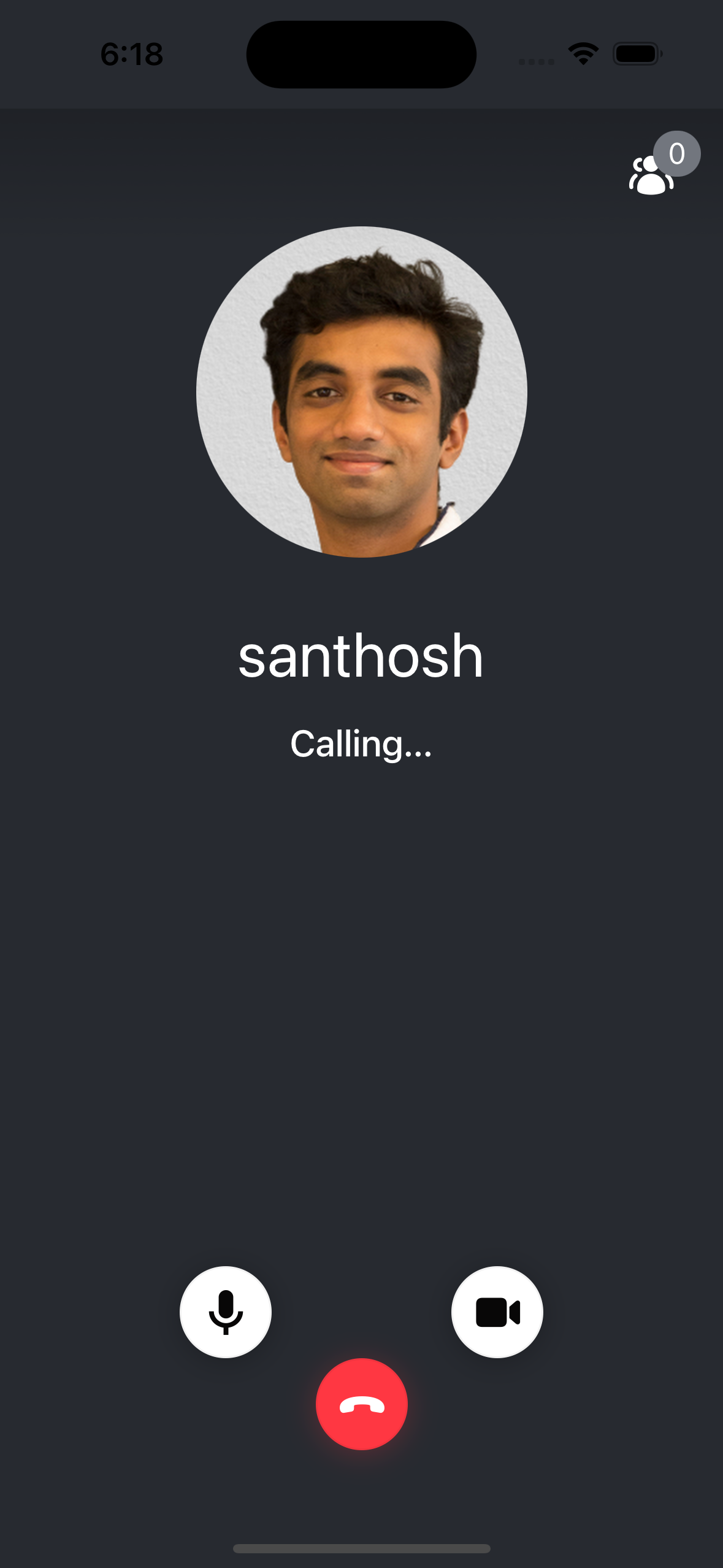
const App = () => {
return (
<View style={styles.container}>
<RingingCallContent />
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
},
});
UI Component Customization
Stream SDK provides highly customizable UI components. Therefore, you can adjust each style or implement your own UI for each part of the components. This list describes what you can do with Stream SDK's UI components:
- You can also build your UI components from scratch with our low-level UI component using our UI Cookbook.
- Use our library of built-in components.
- Mix & Match between your own and built-in components.