Watching a livestream
The Video API allows you to assign specific roles for users in a livestream, such as hosts and viewers. Our SDK provides dedicated livestreaming components for both of these roles.
The ViewerLivestream
component leverages the WebRTC protocol for seamless livestream viewing within the SDK. To enable external publishing, you can access HLS credentials from the dashboard. For additional information, please refer to our livestream tutorial.
This guide describes how to customize watching a livestream through our SDK.
Default component
For the viewer role, our React Native SDK includes the specialized ViewerLivestream
component.
Here is a preview of the above component in video mode:
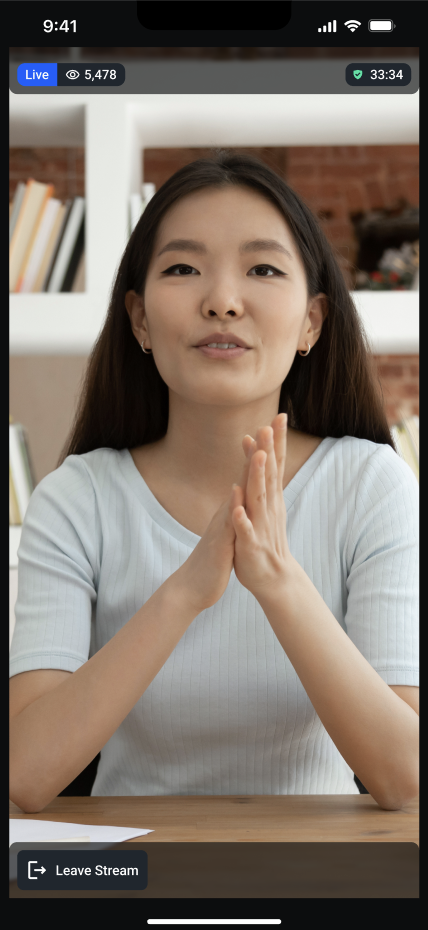
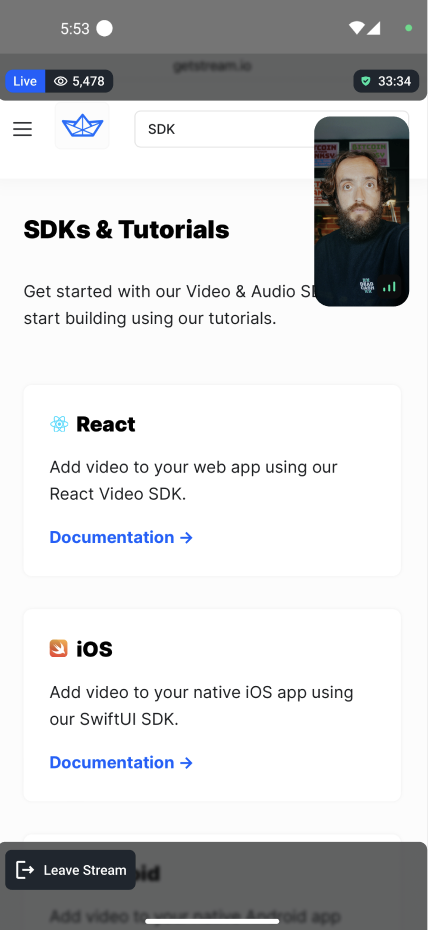
import {
ViewerLivestream,
StreamVideo,
StreamCall,
} from '@stream-io/video-react-native-sdk';
export const MyLivestreamApp = () => {
// init client and call here...
return (
<StreamVideo client={client}>
<StreamCall call={call}>
<ViewerLivestream />
</StreamCall>
</StreamVideo>
);
};
Adding customization
The ViewerLivestream
provides a lot of customization options that can be passed as props:
ViewerLivestreamTopView
allows customizing the top view or the header of theViewerLivestream
. It contains theLiveIndicator
,FollowerCount
, and theDurationBadge
component by default.LivestreamLayout
allows customizing the main video layout component of theViewerLivestream
.ViewerLivestreamControls
allows customizing the bottom livestream controls component of theViewerLivestream
. It contains theViewerLeaveStreamButton
.LiveIndicator
allows customizing the live indicator component that is present in the top view of theViewerLivestream
.FollowerCount
allows customizing the follower count component that is present in the top view of theViewerLivestream
.DurationBadge
allows customizing the duration badge that shows the duration of the livestream in the top view of theViewerLivestream
.ViewerLeaveStreamButton
allows customizing the leave button of the livestream on the controls of theViewerLivestream
.FloatingParticipantView
allows customizing the Floating Participant View that renders the video of the participant when screen is shared.onLeaveStreamHandler
allows full override of the default functionality on what should happen when viewer ends the streaming usingViewerLeaveStreamButton
.
An example to customize the ViewerLeaveStreamButton
button is shown below:
import {
ViewerLivestream,
StreamVideo,
StreamCall,
useCall,
} from '@stream-io/video-react-native-sdk';
import { Button } from 'react-native';
const ViewerLeaveStreamButtonComponent = () => {
const call = useCall();
const onPressHandler = async () => {
await call.leave();
};
return <Button title="Leave Stream" onPress={onPressHandler} />;
};
export const MyLivestreamApp = () => {
// init client and call here...
return (
<StreamVideo client={client}>
<StreamCall call={call}>
<ViewerLivestream
ViewerLeaveStreamButton={ViewerLeaveStreamButtonComponent}
/>
</StreamCall>
</StreamVideo>
);
};
Result: