Participant Label
There may be a plethora of designs for label that is displayed above the participant video. The SDK's default label shows:
- user's name
- microphone and camera mute state
- dominant speaker label
- connection quality indicator
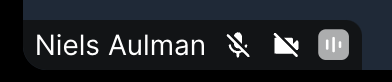
It is expected that the default component may not meet all the requirements of all the apps in the world. Therefore, we will look into ways, how to customize the contents of the participant label in this tutorial.
Custom participant label
Our custom label will include a human-readable call join time in addition to what the design requirements were. To achieve this, we will need to override the whole participantParticipantViewUI
component. You can learn more about ParticipantViewUI
customizations in the participant view customizations guide.
To retrieve the data about the participant, whose view we are about to render, we use useParticipantViewContext
context consumer hook.
import { useParticipantViewContext } from '@stream-io/video-react-sdk';
import { getHumanReadableTimeElapsed } from '../utils';
const ParticipantDetails = () => {
const { participant } = useParticipantViewContext();
const readableTimeSinceJoined = getHumanReadableTimeElapsed(
participant.joinedAt,
);
return (
<div title={participant.name}>
<span>{participant.name}</span>
<span>{readableTimeSinceJoined}</span>
</div>
);
};
export const CustomParticipantViewUI = () => {
return (
<>
<ParticipantDetails />
{/* your other custom UI elements */}
</>
);
};
Final steps
Now we can pass this custom ParticipantViewUI
component down to our call layout components or directly to ParticipantView
component in our custom call layout as described in the aforementioned ParticipantView customizations guide.
import { useCallStateHooks } from '@stream-io/video-react-sdk';
import { CustomParticipantViewUI } from '../ParticipantViewUI';
import { CustomVideoPlaceholder } from '../VideoPlaceholder';
export const CustomCallLayout = () => {
const { useParticipants } = useCallStateHooks();
const participants = useParticipants();
return (
<div>
{/* your other custom UI elements */}
{participants.map((participant) => (
<div key={participant.sessionId}>
<ParticipantView
participant={participant}
ParticipantViewUI={CustomParticipantViewUI}
VideoPlaceholder={CustomVideoPlaceholder}
/>
</div>
))}
</div>
);
};