Build a Swift Chat Messaging App with UIKit
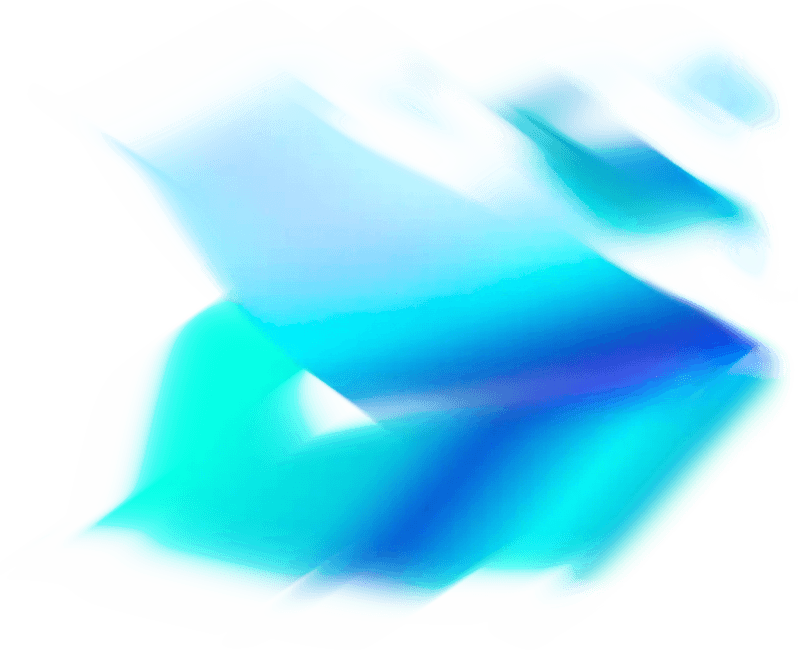
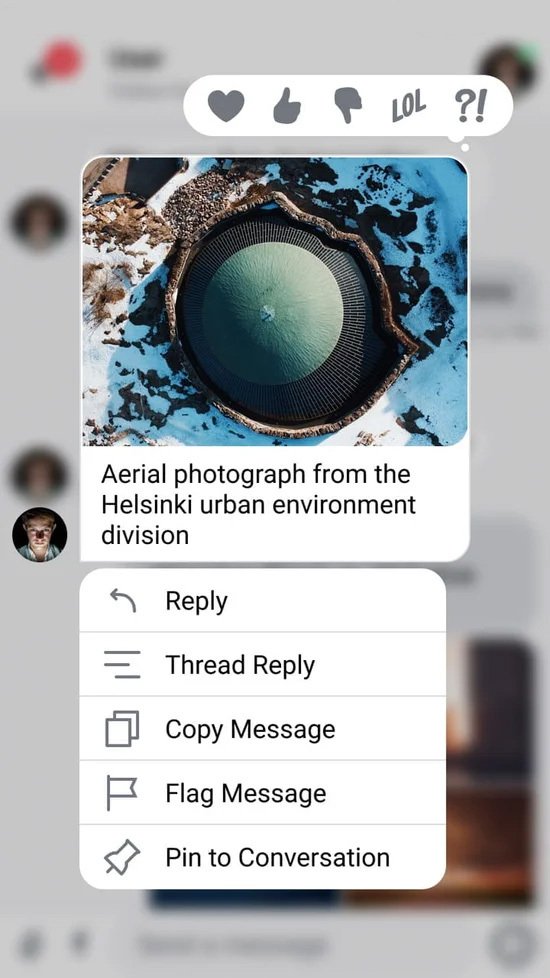
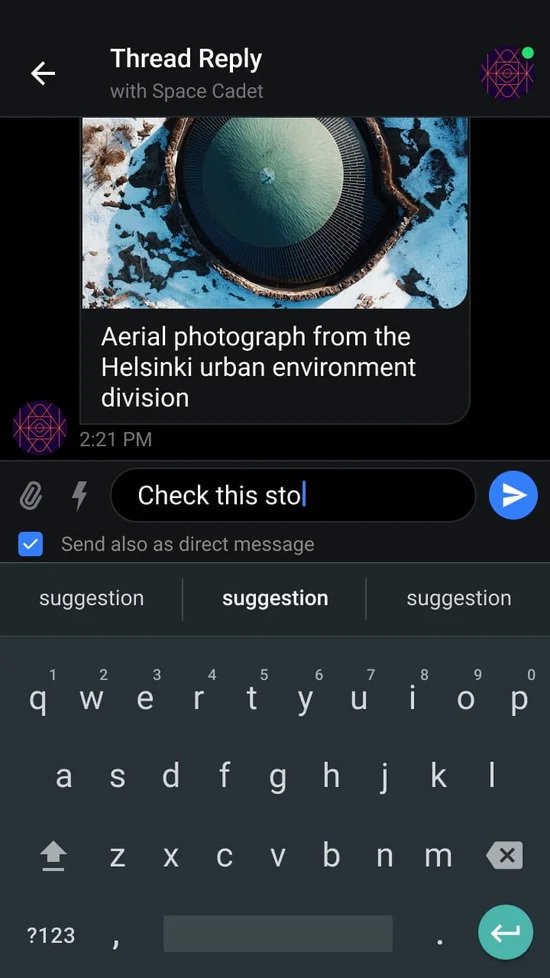
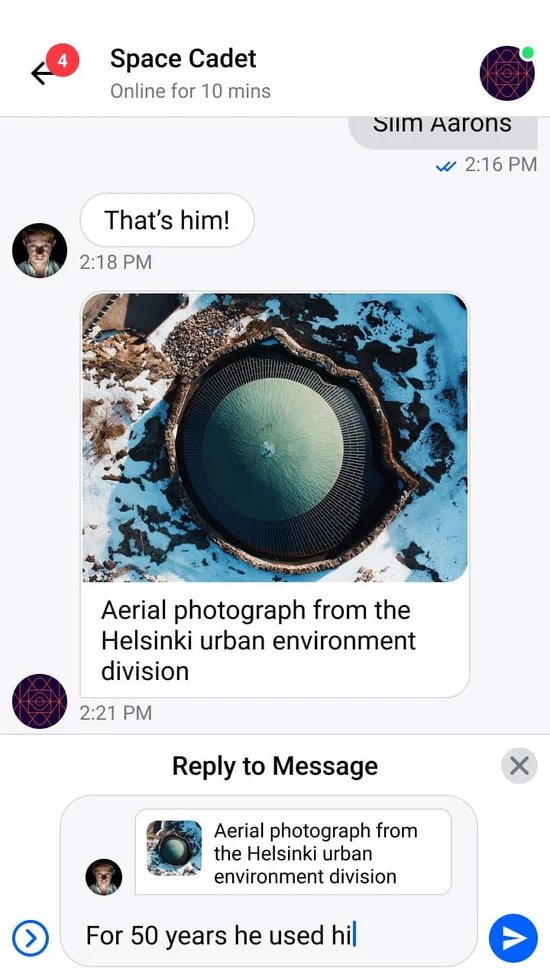
Creating a project
Confused about "Creating a project"?
Let us know how we can improve our documentation:
The completed app for each step of the tutorial is available on GitHub.
To get started with the iOS Chat SDK, open Xcode and create a new project. In this tutorial we are going to create a chat app using UIKit. If you prefer using SwiftUI make sure to look at our SwiftUI tutorial.
- Create a new Xcode project in Xcode (14 or later)
- Choose iOS from the list of platforms
- Choose the App template
- Use "ChatDemo" for the product name
- Select Storyboard in the Interface options
- Make sure Swift is selected as the language option and press "Next" button.
- Select where you want to store your new project and press "Create" button. Your are now ready to start with the tutorial.
In this tutorial we are going to use Swift Package Manager as the dependency manager. The SDK can also be installed with CocoaPods, you can find more information about that on the SDK doc pages.
- Go to File > Add Packages...
- Paste the following URL in the search field at the top right: https://github.com/getstream/stream-chat-swift
- Under Dependency Rule go with the "Up to Next Major Version" option and enter
4.0.0
as the version - Click the Add Package button
- Add both
StreamChat
andStreamChatUI
packages to the project
Displaying a List of Channels
Confused about "Displaying a List of Channels"?
Let us know how we can improve our documentation:
Stream provides a low-level client, offline support, and convenient UI components to help you quickly build your messaging interface. In this section, we'll be using the UI components to quickly display a channel list. The first thing which we will do is to create a globally accessible shared instance of the Stream Chat Client and initialize its connection when the app starts. Next, we will fetch the channels which our user participates in and display it.
First, open the AppDelegate.swift
file and add this extension to ChatClient
at the top of the file:
Next, open ViewController.swift
and change its content to this:
Next, open up SceneDelegate.swift
add import StreamChat
at the top of the file and change the scene(_ scene: UIScene, willConnectTo session: UISceneSession, options connectionOptions: UIScene.ConnectionOptions)
method to the following:
Run the application and you will be able to see a built-in list of channels for the tutorial user, open channels from the list as well and try all built-in chat features.
Let's have a quick look at the different steps in source code shown above:
- We initialize the shared
ChatClient
using an API key. This API key points to a tutorial environment, but you can sign up for a free Chat trial to get your own later. - We create and connect the user using
ChatClient.connectUser
method and use a pre-generated user token, in order to authenticate the user. In a real-world application, your authentication backend would generate such a token at login / signup and hand it over to the mobile app. For more information, see the Tokens & Authentication page. - We use the
DemoChannelList
component and initialize thechannelListController
controller with aChannelListQuery
. We’re using the default sort option which orders the channels bylast_updated_at
time, putting the most recently used channels on the top. For the filter, we’re specifying all channels of typemessaging
where the current user is a member. The documentation about Querying Channels covers this in more detail. - We set the
channelList
as the root of a newUINavigationController
and make it the root of ourwindow
Project Permissions
Before we launch the app, let's add permissions to our app so that we can access the camera and gallery. Our message input UI component supports this by default.
- Select your project from the Project navigator on the left
- Open the Info tab for the ChatDemo target
- Add a new entry with "Privacy - Camera Usage Description" as key and "ChatDemo camera use" as value
- Add a new entry with "Privacy - Photo Library Usage Description" as key and "ChatDemo photo use" as value
Chat Features
Confused about "Chat Features"?
Let us know how we can improve our documentation:
Congrats on getting your chat experience up and running! Stream Chat provides you with all the features you need to build an engaging messaging experience:
- Offline support: send messages, edit messages and send reactions while offline
- Link previews: generated automatically when you send a link
- Commands: type
/
to use commands like/giphy
- Reactions: long-press on a message to add a reaction
- Attachments: use the paperclip button in
MessageInputView
to attach images and files - Edit message: long-press on your message for message options, including editing
- Threads: start message threads to reply to any message
The Stream messaging API is powered by Go, RocksDB and Raft. The API tends to respond in less than 10ms and powers activity feeds and chat for over a billion end users.
Some features are hard to see in action with just one user online. You can open the same channel on the web and try user-to-user interactions like typing events, reactions, and threads.
Chat Message Customization
Confused about "Chat Message Customization"?
Let us know how we can improve our documentation:
Next we will create a DemoChannelVC
view controller so that we can show how you can customize the channel screen.
Under File > New > File > Cocoa Touch Class we create a new file called "DemoChannelVC", open it in our editor and make it inherit from ChatChannelVC
.
Open the SceneDelegate
and make these two changes:
- Append this at the top after the other imports
- Call
applyChatCustomizations
right before the initialization ofChatClient
Let's now look at how we can customize the chat experience:
- Change message styles using attributes
- Create a custom attachment view
- Build your own views on top of the controllers provided by
StreamChat
The first customization is fairly simple, components expose many attributes to handle the most common cases. Changing colors, font and images is very simple. The SDK allows you to make theming changes using the Appearance.default
object.
To see this in practice, go back to SceneDelegate.swift
and update the applyChatCustomizations
function to looks like this:
The Appearance
object exposes all colors, fonts and images used by components. This makes it very easy to apply theme changes consistently across the entire application. Similar to Components
you need to make changes to theming as early as possible in the application life cycle.
Before moving to the next section, let's look at how we added the channel screen to the application:
- First we added the channel list view controller with a navigation controller. This allows the SDK to navigate from the channel list to the channel screen.
- We added a new view controller and subclassed
ChatChannelVC
the VC that provides the channel functionality - We configured StreamChat to use our
DemoChannelVC
class. The SDK exposes most of the UI configuration on theComponents.default
object. These kind of changes are best done when the application is starting.
Creating Custom Attachment Views
Confused about "Creating Custom Attachment Views"?
Let us know how we can improve our documentation:
There may come a time when you have requirements to include things in your chat experience that we don't provide out-of-the-box. For times like this, we provide two main customization paths: you can either reimplement the entire message component and display a message how you like, or you can use custom attachment views. We'll look at this latter approach now.
You could use this to embed a shopping cart in your chat, share a location, or perhaps implement a poll. For this example, we'll keep it simple and customize the preview for images shared from Imgur. We're going to render the Imgur logo over images from the imgur.com domain.
Download the Imgur logo and drop the file inside Assets.xcassets
using Xcode.
Also, for this example we will be using Nuke as our Image loading system dependency. You can add it to your project by going to File > Add Packages..., pasting the following URL in the search field at the top right: https://github.com/kean/Nuke and pressing "Add Package" button.
After that we are going to do the following:
- Create a custom view to display the image, and the Imgur logo overlay
- Create the
AttachmentInjectorView
class that adds our custom view to the message layout and initialize it with the content - Create a custom
AttachmentViewCatalog
class, this is the class that is responsible for selecting the right view for a message attachment - Register the custom catalog class to the
Components
object
The Custom Attachment View
You can place this code in a new file or simply add it to ViewController.swift
Here we added a UIView class called ImgurImageAttachmentView
, this view class exposes a computed property content
to hold and represent the attachment. Most of the code is just UIKit boilerplate code to render the labels and the Imgur logo on top of the image attachment.
The Custom AttachmentInjectorView
You can place this code right after the ImgurImageAttachmentView
code.
The ImgurImageAttachmentViewInjector
is a subclass of AttachmentViewInjector
and wraps our custom view class. The two life-cycle methods contentViewDidLayout
and contentViewDidUpdateContent
are called by the SDK, this is where we set up our custom class with the right layout and with the content.
The Custom AttachmentViewCatalog
You can place this code right after the ImgurImageAttachmentViewInjector
code.
The SDK uses the AttachmentViewCatalog
to pick the appropriate AttachmentViewInjector
for each message's attachments. Our subclass first checks if the message has any link attachment with images coming from the 'imgur.com' host and returns the custom injector for them.
Lastly, you need to configure the SDK to use the MyAttachmentViewCatalog
. To do this you need to update the applyChatCustomizations
function from SceneDelegate.swift
.
When you run your app, you should now see the Imgur logo displayed over images from Imgur. You can test this by posting an Imgur link like this one: https://imgur.com/gallery/ro2nIC6.
This was, of course, a very simple change, but you could use the same approach to implement a product preview, shopping cart, location sharing, polls, and more. You can achieve lots of your message customization goals by implementing a custom attachment View.
If you need even more customization, you can also implement custom message views for the entire message object.
Creating a Typing Status Component
Confused about "Creating a Typing Status Component"?
Let us know how we can improve our documentation:
The channel list component shows typing indicators out of the box, in this example we are going to handle typing events ourselves and show our own.
The ChatChannelVC
class is a delegate of the ChatChannelController
controller and conforms to the ChatChannelControllerDelegate
protocol.
This protocol allows delegates to receive channel updates including changes to the list of typing users, the latter via this method:
Now that we know this, we can override the implementation from ChatChannelVC
and write our own. This is how the DemoChannelVC.swift
file should look like:
You can open the same channel on the web and try this using a different user.
Enabling Console Logs
Confused about "Enabling Console Logs"?
Let us know how we can improve our documentation:
By default console logs are disabled. But it's likely whilst you're building your app that you will need to enable logs at some point.
Let's go ahead and enable your console logs now.
By setting your log level to .info
means you will get access to all console logs provided by the SDK.
The SDK supports many more logs, and you can read more about them here.
Congratulations!
Confused about "Congratulations!"?
Let us know how we can improve our documentation:
In this iOS in-app messaging tutorial, you learned how to build a fully functional chat app with Swift and UIKit. You also learned how easy it is to customize the behavior and build any type of chat or messaging experience.
Remember, you can also check out the completed app for the tutorial on GitHub.
If you want to get started on integrating chat into your own messaging app, sign up for a free Chat trial, and get your own API key to build with!
To recap, our iOS Chat SDK consists of two frameworks which give you an opportunity to interact with Stream Chat APIs on a different level:
- StreamChat - The official low-level Swift SDK for Stream Chat. It allows you to make API calls and receive events whenever something changes on a user or channel that you’re watching.
- StreamChatUI - Builds on top of the low level client and provides fully custom UI, this library is your best starting point.
The underlying chat API is based on Go, RocksDB, and Raft. This makes the in-app chat experience extremely fast with response times that are often below 10ms.
Final Thoughts
In this chat app tutorial we built a fully functioning iOS messaging app with our SDK component library. We also showed how easy it is to customize the behavior and the style of the iOS chat app components with minimal code changes.
Both the chat SDK for iOS and the API have plenty more features available to support more advanced use-cases such as push notifications, content moderation, rich messages and more. You may also want to read our SwiftUI tutorial too.
Give us Feedback!
Did you find this tutorial helpful in getting you up and running with iOS for adding chat to your project? Either good or bad, we’re looking for your honest feedback so we can improve.
We’ve been going at lightspeed to build more communication functionality into Kiddom. The only way to achieve this in four months was to do a chat integration with Stream because we needed to do it reliably and at scale.
Nick Chen
Head of Product, Kiddom