Messages Overview
Confused about "Messages Overview"?
Let us know how we can improve our documentation:
Let's dive right into it, the example below shows how to send a simple message using Stream:
Note how server side SDKs require that you specify user_id to indicate who is sending the message. You can add custom fields to both the message and the attachments. There's a 5KB limit for the custom fields. File uploads are uploaded to the CDN so don't count towards this 5KB limit.
name | type | description | default | optional |
---|---|---|---|---|
text | string | The text of the chat message (Stream chat supports markdown and automatically enriches URLs). | ✓ | |
attachments | array | A list of attachments (audio, videos, images, and text). Max is 30 attachments per message. The total combined attachment size can't exceed 5KB. | ||
user_id | object | This value is automatically set in client-side mode. You only need to send this value when using the server-side APIs. | ✓ | |
mentioned_users | array | A list of users mentioned in the message. You send this as a list of user IDs and receive back the full user data. | ||
message custom data | object | Extra data for the message. Must not exceed 5KB in size. | ||
skip_push | bool | do not send a push notification | false | ✓ |
Complex Example
Copied!Confused about "Complex Example"?
Let us know how we can improve our documentation:
A more complex example for creating a message is shown below:
By default Stream’s UI components support the following attachment types:
- Audio
- Video
- Image
- Text
You can specify different types as long as you implement the frontend rendering logic to handle them. Common use cases include:
- Embedding products (photos, descriptions, outbound links, etc.)
- Sharing of a users location
The React tutorial for Stream Chat explains how to customize the Attachment
component.
Get a Message
Copied!Confused about "Get a Message"?
Let us know how we can improve our documentation:
You can get a single message by its ID using the getMessage
call:
Get a Message Options
Copied!Confused about "Get a Message Options"?
Let us know how we can improve our documentation:
name | type | description | default | optional |
---|---|---|---|---|
show_deleted_message | boolean | if true, returns the original message | false | ✓ |
Update a Message
Copied!Confused about "Update a Message"?
Let us know how we can improve our documentation:
You can edit a message by calling updateMessage
and including a message with an ID – the ID field is required when editing a message:
Partial Update
Copied!Confused about "Partial Update"?
Let us know how we can improve our documentation:
A partial update can be used to set and unset specific fields when it is necessary to retain additional data fields on the object. AKA a patch style update.
Delete A Message
Copied!Confused about "Delete A Message"?
Let us know how we can improve our documentation:
You can delete a message by calling deleteMessage
and including a message with an ID. Messages can be soft deleted or hard deleted. Unless specified via the hard
parameter, messages are soft deleted. Be aware that deleting a message doesn't delete its attachments. See the docs for more information on deleting attachments.
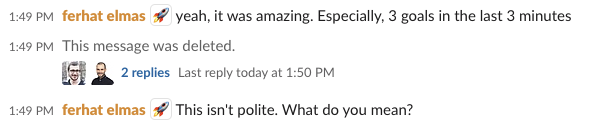
Soft delete
Copied!Can be done client-side by users
Message is still returned in the message list and all its data is kept as it is
Message type is set to "deleted"
Reactions and replies are kept in place
Can be undeleted
Hard delete
Copied!Can be done client-side by users but be cautious this action is not recoverable
The message is removed from the channel and its data is wiped
All reactions are deleted
All replies and their reactions are deleted
By default messages are soft deleted, this is a great way to keep the channel history consistent.
Undelete a message
Copied!Confused about "Undelete a message"?
Let us know how we can improve our documentation:
A message that was soft-deleted can be undeleted. This is only allowed for server-side clients. The userID
specifies the user that undeleted the message, which can be used for auditing purposes.
Messages can be undeleted if:
The message was soft-deleted
The channel has not been deleted
It is not a reply to a deleted message. If it is, the parent must be undeleted first
The user that undeletes the message is valid